Screen Share - Android
This feature enables hosts to start or stop sharing their screen with other hosts and audience members during the live stream. Only hosts (in SEND_AND_RECV
mode) can broadcast their screen, while audience members (in RECV_ONLY
mode) can view it in real time.
How Screen share works?​
- The following diagram shows flow of the screen sharing in android using VideoSDK :
enableScreenShare()
​
-
By using
enableScreenShare()
function ofMeeting
class,the host can share their mobile screen to other hosts and audience members. -
You can pass customised screenshare track in
enableScreenShare()
by using Custom Screen Share Track. -
Screen Share stream of the participant can be accessed from the
onStreamEnabled
event ofParticipantEventListener
.
Screenshare permission​
-
A participant’s Screen share stream is provided via the
MediaProjection
API. This API is only compatible withBuild.VERSION_CODES.LOLLIPOP
or higher. -
Get an instance of the
MediaProjectionManager
and Call thecreateScreenCaptureIntent()
method in an activity. This initiates a prompt dialog for the user to confirm screen projection. -
One will get a prompt dialog like this:
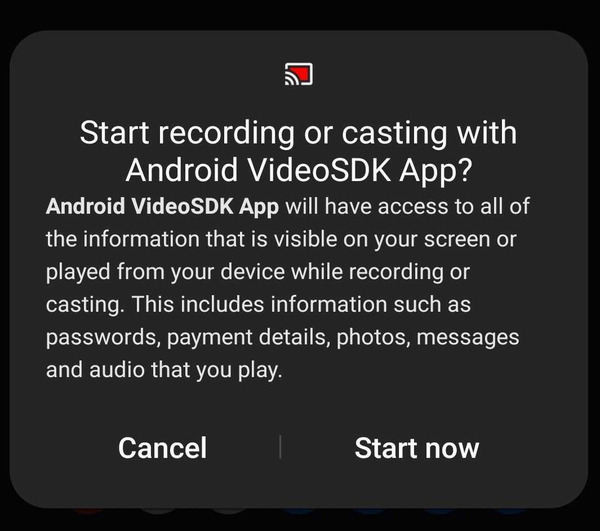
- After permission is received from the user, you can call
enableScreenShare()
method.
- Kotlin
- Java
private fun enableScreenShare() {
val mediaProjectionManager = application.getSystemService(
MEDIA_PROJECTION_SERVICE
) as MediaProjectionManager
startActivityForResult(
mediaProjectionManager.createScreenCaptureIntent(), CAPTURE_PERMISSION_REQUEST_CODE
)
}
public override fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent?) {
super.onActivityResult(requestCode, resultCode, data)
if (requestCode != CAPTURE_PERMISSION_REQUEST_CODE) return
if (resultCode == RESULT_OK) {
// Enabling screen share
liveStream!!.enableScreenShare(data)
}
}
private void enableScreenShare() {
MediaProjectionManager mediaProjectionManager =
(MediaProjectionManager) getApplication().getSystemService(
Context.MEDIA_PROJECTION_SERVICE);
startActivityForResult(
mediaProjectionManager.createScreenCaptureIntent(), CAPTURE_PERMISSION_REQUEST_CODE);
}
@Override
public void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode != CAPTURE_PERMISSION_REQUEST_CODE)
return;
if (resultCode == Activity.RESULT_OK) {
// Enabling screen share
liveStream.enableScreenShare(data);
}
}
Customise notification​
-
When a presenter starts screen share, presenter will receive a notification with a pre-defined title and message.
-
Notification with pre-defined title and message will look like this:
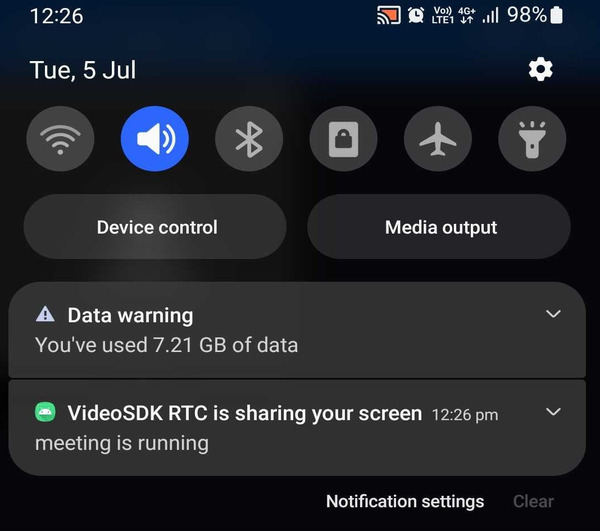
- You can Customise those title, message and icon as per your requirements using
<meta-data>
specified inapp/src/main/AndroidManifest.xml
.
<application>
<meta-data
android:name="notificationTitle"
android:value="@string/notificationTitle"
/>
<meta-data
android:name="notificationContent"
android:value="@string/notificationContent"
/>
<meta-data
android:name="notificationIcon"
android:resource="@mipmap/ic_launcher_round"
/>
</application>
disableScreenShare()
​
- By using
disableScreenShare()
function ofMeeting
class,the host can stop sharing their mobile screen to other hosts and audience members.
- Kotlin
- Java
private fun disableScreenShare() {
// Disabling screen share
liveStream!!.disableScreenShare()
}
private void disableScreenShare(){
// Disabling screen share
liveStream.disableScreenShare();
}
Events associated with enableScreenShare​
-
Participant who share their mobile screen will receive a callback on
onStreamEnabled()
of theParticipant
withStream
object. -
While other Participants will receive
onPresenterChanged()
callback of theMeeting
class with the participantId aspresenterId
who started the screen share.
Events associated with disableScreenShare​
-
Participant who shared their mobile screen will receive a callback on
onStreamDisabled()
of theParticipant
withStream
object. -
While other Participants will receive
onPresenterChanged()
callback of theMeeting
class with thepresenterId
asnull
indicating there is no presenter.
- Kotlin
- Java
private fun setLocalListeners() {
liveStream!!.localParticipant.addEventListener(object : ParticipantEventListener() {
//Callback for when the participant starts a stream
override fun onStreamEnabled(stream: Stream) {
if (stream.kind.equals("share", ignoreCase = true)) {
Log.d("VideoSDK","Share Stream On: onStreamEnabled $stream");
}
}
//Callback for when the participant stops a stream
override fun onStreamDisabled(stream: Stream) {
if (stream.kind.equals("share", ignoreCase = true)) {
Log.d("VideoSDK","Share Stream On: onStreamDisabled $stream");
}
}
});
}
private val meetingEventListener: MeetingEventListener = object : MeetingEventListener() {
//Callback for when the presenter changes
override fun onPresenterChanged(participantId: String) {
if(!TextUtils.isEmpty(participantId))
{
Log.d("VideoSDK","$participantId started screen share");
}else{
Log.d("VideoSDK","some one stopped screen share");
}
}
}
private void setLocalListeners() {
liveStream.getLocalParticipant().addEventListener(new ParticipantEventListener() {
//Callback for when the participant starts a stream
@Override
public void onStreamEnabled(Stream stream) {
if (stream.getKind().equalsIgnoreCase("share")) {
Log.d("VideoSDK","Share Stream On: onStreamEnabled" + stream);
}
}
//Callback for when the participant stops a stream
@Override
public void onStreamDisabled(Stream stream) {
if (stream.getKind().equalsIgnoreCase("share")) {
Log.d("VideoSDK","Share Stream Off: onStreamDisabled" + stream);
}
}
});
}
private final MeetingEventListener meetingEventListener = new MeetingEventListener() {
//Callback for when the presenter changes
@Override
public void onPresenterChanged(String participantId) {
if(participantId != null){
Log.d("VideoSDK",participantId + "started screen share");
}else{
Log.d("VideoSDK","some one stopped screen share");
}
}
};
Rendering Screen Share Stream​
- When a host participant shares their screen, the
onStreamEnabled()
function of theParticipantEventListener
is triggered, allowing theStream
to be added to aVideoView
.
- Kotlin
- Java
private fun setLocalListeners() {
liveStream!!.localParticipant.addEventListener(object : ParticipantEventListener() {
override fun onStreamEnabled(stream: Stream) {
if (stream.kind.equals("share", ignoreCase = true)) {
// display share video
val videoTrack = stream.track as VideoTrack
shareView!!.addTrack(videoTrack)
}
}
override fun onStreamDisabled(stream: Stream) {
if (stream.kind.equals("share", ignoreCase = true)) {
shareView!!.removeTrack()
}
}
});
}
private void setLocalListeners() {
liveStream.getLocalParticipant().addEventListener(new ParticipantEventListener() {
@Override
public void onStreamEnabled(Stream stream) {
if (stream.getKind().equalsIgnoreCase("share")) {
// display share video
VideoTrack videoTrack = (VideoTrack) stream.getTrack();
shareView.addTrack(videoTrack);
}
}
@Override
public void onStreamDisabled(Stream stream) {
if (stream.getKind().equalsIgnoreCase("share")) {
shareView.removeTrack();
}
}
});
}
API Reference​
The API references for all the methods and events utilised in this guide are provided below.
Got a Question? Ask us on discord