Share Your Screen - Android
-
Whenever any participant wants to share a complete screen of mobile, they can simply do it with VideoSDK Meeting.
-
This guide will provide an overview of how to enable or disable Screen Share in a meeting.
How Screen share works?​
- The following diagram shows flow of the screen sharing in android using VideoSDK:
Enable Screen Share​
-
A participant’s Screen share stream is provided via the
MediaProjection
API. This API is only compatible withBuild.VERSION_CODES.LOLLIPOP
or higher. -
Get an instance of the
MediaProjectionManager
and Call thecreateScreenCaptureIntent()
method in an activity. This initiates a prompt dialog for the user to confirm screen projection. -
One will get a prompt dialog like this:
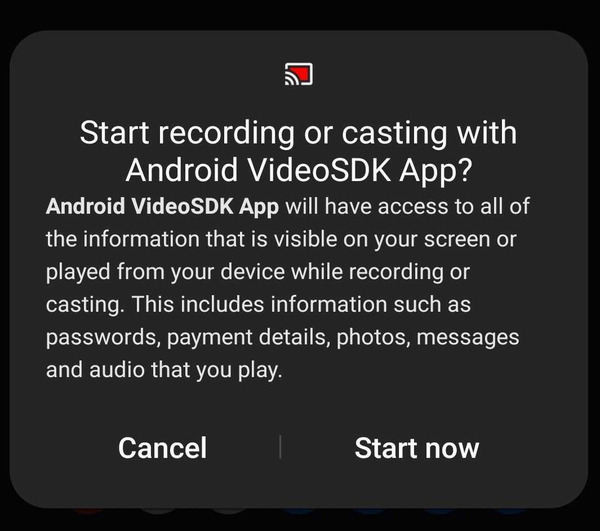
- After permission is received from the user, call
meeting.enableScreenShare()
method.
- Kotlin
- Java
private fun enableScreenShare() {
val mediaProjectionManager = application.getSystemService(
MEDIA_PROJECTION_SERVICE
) as MediaProjectionManager
startActivityForResult(
mediaProjectionManager.createScreenCaptureIntent(), CAPTURE_PERMISSION_REQUEST_CODE
)
}
public override fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent?) {
super.onActivityResult(requestCode, resultCode, data)
if (requestCode != CAPTURE_PERMISSION_REQUEST_CODE) return
if (resultCode == RESULT_OK) {
meeting!!.enableScreenShare(data)
}
}
private void enableScreenShare() {
MediaProjectionManager mediaProjectionManager =
(MediaProjectionManager) getApplication().getSystemService(
Context.MEDIA_PROJECTION_SERVICE);
startActivityForResult(
mediaProjectionManager.createScreenCaptureIntent(), CAPTURE_PERMISSION_REQUEST_CODE);
}
@Override
public void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode != CAPTURE_PERMISSION_REQUEST_CODE)
return;
if (resultCode == Activity.RESULT_OK) {
meeting.enableScreenShare(data);
}
}
Customise notification​
-
When a presenter starts screen share, presenter will receive a notification with a pre-defined title and message.
-
Notification with pre-defined title and message will look like this:
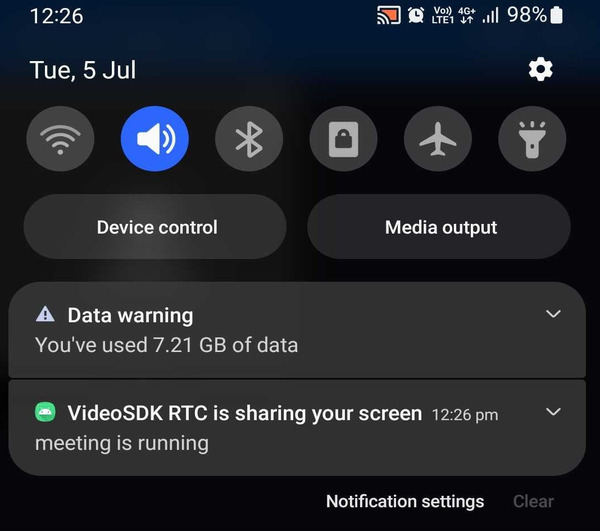
- You can Customise those title, message and icon as per your requirements using
<meta-data>
specified inapp/src/main/AndroidManifest.xml
.
<application>
<meta-data
android:name="notificationTitle"
android:value="@string/notificationTitle"
/>
<meta-data
android:name="notificationContent"
android:value="@string/notificationContent"
/>
<meta-data
android:name="notificationIcon"
android:resource="@mipmap/ic_launcher_round"
/>
</application>
Disable Screen Share​
By using meeting.disableScreenShare()
function, a participant can stop publishing screen stream to other participants.
- Kotlin
- Java
private fun disableScreenShare() {
meeting!!.disableScreenShare()
}
private void disableScreenShare(){
meeting.disableScreenShare();
}
Display Screen Share Stream​
Local Participant​
When a Local participant share the screen, onStreamEnabled()
of ParticipantEventListener
is triggered with the Stream
which can be added to a VideoView
.
- Kotlin
- Java
private fun setLocalListeners() {
meeting!!.localParticipant.addEventListener(object : ParticipantEventListener() {
override fun onStreamEnabled(stream: Stream) {
if (stream.kind.equals("share", ignoreCase = true)) {
// display share video
val videoTrack = stream.track as VideoTrack
shareView!!.addTrack(videoTrack)
}
}
override fun onStreamDisabled(stream: Stream) {
if (stream.kind.equals("share", ignoreCase = true)) {
shareView!!.removeTrack()
}
}
});
}
private void setLocalListeners() {
meeting.getLocalParticipant().addEventListener(new ParticipantEventListener() {
@Override
public void onStreamEnabled(Stream stream) {
if (stream.getKind().equalsIgnoreCase("share")) {
// display share video
VideoTrack videoTrack = (VideoTrack) stream.getTrack();
shareView.addTrack(videoTrack);
}
}
@Override
public void onStreamDisabled(Stream stream) {
if (stream.getKind().equalsIgnoreCase("share")) {
shareView.removeTrack();
}
}
});
}
Other Participants​
When other participant(Except you) share their screen, onPresenterChanged()
in the MeetingEventListener
is triggered with the participantId
of the screen share.
- Kotlin
- Java
private val meetingEventListener: MeetingEventListener = object : MeetingEventListener() {
override fun onPresenterChanged(participantId: String) {
updatePresenter(participantId)
}
}
//Getting the stream from the participantId
private fun updatePresenter(participantId: String?) {
// find participant
val participant = meeting!!.participants.get(participantId) ?: return
// find share stream in participant
var shareStream: Stream? = null
for (stream: Stream in participant.streams.values) {
if ((stream.kind == "share")) {
shareStream = stream
break
}
}
if (shareStream == null) return
// display share video
val videoTrack = shareStream.track as VideoTrack
shareView!!.addTrack(videoTrack)
// listen for share stop event
participant.addEventListener(object : ParticipantEventListener() {
override fun onStreamDisabled(stream: Stream) {
if ((stream.kind == "share")) {
shareView!!.removeTrack()
}
}
})
}
private final MeetingEventListener meetingEventListener = new MeetingEventListener() {
//Triggered when Presenter changes
@Override
public void onPresenterChanged(String participantId) {
updatePresenter(participantId);
}
};
//Getting the stream from the participantId
private void updatePresenter(String participantId) {
// find participant
Participant participant = meeting.getParticipants().get(participantId);
if (participant == null) return;
// find share stream in participant
Stream shareStream = null;
for (Stream stream: participant.getStreams().values()) {
if (stream.getKind().equals("share")) {
shareStream = stream;
break;
}
}
if (shareStream == null) return;
// display share video
VideoTrack videoTrack = (VideoTrack) shareStream.getTrack();
shareView.addTrack(videoTrack)
// listen for share stop event
participant.addEventListener(new ParticipantEventListener() {
@Override
public void onStreamDisabled(Stream stream) {
if (stream.getKind().equals("share")) {
shareView.removeTrack();
}
}
});
}
- Here screenShare stream is displayed using
VideoView
, but you may also useSurfaceViewRender
for the same. - For
VideoView
, SDK version should be0.1.13
or higher. - To know more about
VideoView
, please visit here
Got a Question? Ask us on discord