Start or Join Meeting - Android
After the successful installation of VideoSDK, the next step is to integrate VideoSDK features with your webApp/MobileApp.
To Communicate with other participant's audio or video call, you will need to join the meeting.
This guide will provide an overview of how to configure, initialize and join a VideoSDK meeting.
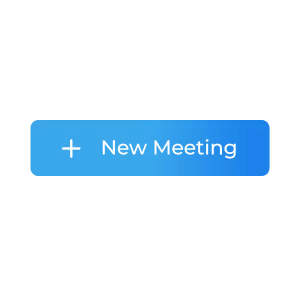
1. Configuration
To configure a meeting, you will need generated token and meetingId, we had discussed in Server Setup. This code snippet calls API from local server
Scenario 1 - Suppose you don't have any meetingId, you can simply generate meetingId by invoking create-meeting
API.
Scenario 2 - Suppose you have meetingId, now you don't have to call create-meeting
API to generate meetingId, instead you can call validate-meeting
API to validate meetingId.
Token generation API is necessary for both scenario.
- Kotlin
- Java
package live.videosdk.rtc.android.java;
import com.androidnetworking.AndroidNetworking;
import com.androidnetworking.error.ANError;
import com.androidnetworking.interfaces.JSONObjectRequestListener;
import org.json.JSONException;
import org.json.JSONObject;
class JoinActivity : AppCompatActivity() {
private val apiServerUrl = "http://localhost:9000"
// ...
// onCreate() and other methods
private fun getToken(@Nullable meetingId: String?) {
AndroidNetworking
.get("$apiServerUrl/get-token")
.build()
.getAsJSONObject(
object : JSONObjectRequestListener {
override fun onResponse(response: JSONObject) {
try
{
token = response.getString("token")
if (meetingId == null) {
createMeeting(token)
} else {
joinMeeting(token, meetingId)
}
} catch (e: JSONException) {
e.printStackTrace()
}
}
override fun onError(anError: ANError) {
anError.printStackTrace()
}
}
)
}
private fun createMeeting(token: String) {
AndroidNetworking
.post("$apiServerUrl/create-meeting")
.addBodyParameter("token", token)
.build()
.getAsJSONObject(
object : JSONObjectRequestListener {
override fun onResponse(response: JSONObject) {
try {
// final String meetingId = response.getString("meetingId");
// Intent intent = new Intent(JoinActivity.this, MainActivity.class);
// intent.putExtra("token", token);
// intent.putExtra("meetingId", meetingId);
// startActivity(intent);
} catch (e: JSONException) {
e.printStackTrace()
}
}
override fun onError(anError: ANError) {
anError.printStackTrace()
}
}
)
}
private fun joinMeeting(token: String, meetingId: String) {
AndroidNetworking
.post("$apiServerUrl/validate-meeting/{meetingId}")
.addPathParameter("meetingId", meetingId)
.addBodyParameter("token", token)
.build()
.getAsJSONObject(
object : JSONObjectRequestListener {
override fun onResponse(response: JSONObject) {
// Intent intent = new Intent(JoinActivity.this, MainActivity.class);
// intent.putExtra("token", token);
// intent.putExtra("meetingId", meetingId);
// startActivity(intent);
}
override fun onError(anError: ANError) {
anError.printStackTrace()
}
}
)
}
}
package live.videosdk.rtc.android.java;
import com.androidnetworking.AndroidNetworking;
import com.androidnetworking.error.ANError;
import com.androidnetworking.interfaces.JSONObjectRequestListener;
import org.json.JSONException;
import org.json.JSONObject;
public class JoinActivity extends AppCompatActivity {
private String apiServerUrl = "http://localhost:9000";
// ...
// onCreate() and other methods
private void getToken(@Nullable String meetingId) {
AndroidNetworking
.get(apiServerUrl + "/get-token")
.build()
.getAsJSONObject(
new JSONObjectRequestListener() {
@Override
public void onResponse(JSONObject response) {
try {
String token = response.getString("token");
if (meetingId == null) {
createMeeting(token);
} else {
joinMeeting(token, meetingId);
}
} catch (JSONException e) {
e.printStackTrace();
}
}
@Override
public void onError(ANError anError) {
anError.printStackTrace();
}
}
);
}
private void createMeeting(String token) {
AndroidNetworking
.post(apiServerUrl + "/create-meeting")
.addBodyParameter("token", token)
.build()
.getAsJSONObject(
new JSONObjectRequestListener() {
@Override
public void onResponse(JSONObject response) {
try {
// final String meetingId = response.getString("meetingId");
// Intent intent = new Intent(JoinActivity.this, MainActivity.class);
// intent.putExtra("token", token);
// intent.putExtra("meetingId", meetingId);
// startActivity(intent);
} catch (JSONException e) {
e.printStackTrace();
}
}
@Override
public void onError(ANError anError) {
anError.printStackTrace();
}
}
);
}
private void joinMeeting(String token, String meetingId) {
AndroidNetworking
.post(apiServerUrl + "/validate-meeting/{meetingId}")
.addPathParameter("meetingId", meetingId)
.addBodyParameter("token", token)
.build()
.getAsJSONObject(
new JSONObjectRequestListener() {
@Override
public void onResponse(JSONObject response) {
// Intent intent = new Intent(JoinActivity.this, MainActivity.class);
// intent.putExtra("token", token);
// intent.putExtra("meetingId", meetingId);
// startActivity(intent);
}
@Override
public void onError(ANError anError) {
anError.printStackTrace();
}
}
);
}
}
2. Initialization
Important Changes Android SDK in Version v0.2.0
- The following modes have been deprecated:
CONFERENCE
has been replaced bySEND_AND_RECV
VIEWER
has been replaced bySIGNALLING_ONLY
Please update your implementation to use the new modes.
⚠️ Compatibility Notice:
To ensure a seamless meeting experience, all participants must use the same SDK version.
Do not mix version v0.2.0 + with older versions, as it may cause significant conflicts.
After configuration, you will have to Initialize
meeting by providing name, meetingId, micEnabled, webcamEnabled & maxResolution.
NOTE : For React & React native developer, you have
to be familiar with hooks concept. You can understand hooks concept on React Hooks.
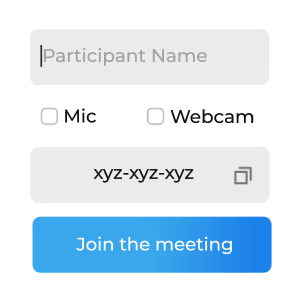
- Kotlin
- Java
import live.videosdk.rtc.android.VideoSDK;
import live.videosdk.rtc.android.Meeting;
class MainActivity : AppCompatActivity() {
private var meeting: Meeting? = null
override fun onCreate(savedInstanceState: Bundle?) {
// Configure parameters
val token = intent.getStringExtra("token")
val meetingId = intent.getStringExtra("meetingId")
val participantName = "John Doe"
val micEnabled = true
val webcamEnabled = true
val paticipantId="demo@123" // If you passed `null` then SDK will create an Id by itself and will use that id.
val mode="SEND_AND_RECV"
// Configure authentication token
VideoSDK.config(token)
// create a new meeting instance
meeting = VideoSDK.initMeeting(
this@MainActivity,
meetingId,
participantName,
micEnabled,
webcamEnabled,
paticipantId,
mode,
null
)
}
}
import live.videosdk.rtc.android.VideoSDK;
import live.videosdk.rtc.android.Meeting;
public class MainActivity extends AppCompatActivity {
private Meeting meeting;
@Override
protected void onCreate(Bundle savedInstanceState) {
// Configure parameters
final String token = getIntent().getStringExtra("token");
final String meetingId = getIntent().getStringExtra("meetingId");
final String participantName = "John Doe";
final boolean micEnabled = true;
final boolean webcamEnabled = true;
final String participantId = "demo@123"; // If you passed `null` then SDK will create an Id by itself and will use that id.
final String mode="SEND_AND_RECV";
// Configure authentication token
VideoSDK.config(token);
// create a new meeting instance
meeting = VideoSDK.initMeeting(
MainActivity.this,
meetingId,
participantName,
micEnabled,
webcamEnabled,
participantId,
mode,
null
);
}
}
3. Join
After configuration & initialization, the third step is to call join() to join a meeting.
After joining, you will be able to Manage Participant in a meeting.
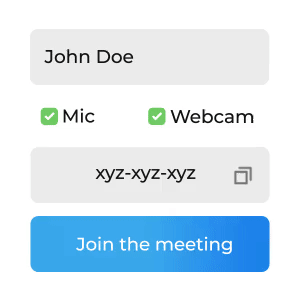
- Kotlin
- Java
// After receiving mic and webcam access permissions
// join the meeting
meeting!!.join()
// After receiving mic and webcam access permissions
// join the meeting
meeting.join();
Events
Following events are emitted on the meeting
when it is successfully joined.
- Local Participant will receive a
onMeetingJoined
event when successfully joined. - Remote Participant will receive a
onParticipantJoined
event with the newly joinedParticipant
object from the event callback.
- Kotlin
- Java
private final MeetingEventListener meetingEventListener = new MeetingEventListener() {
override fun onMeetingJoined() {
Log.d("#meeting", "onMeetingJoined()")
}
override fun onParticipantJoined(participant: Participant) {
Log.d("#meeting", participant.displayName + " left");
}
}
private final MeetingEventListener meetingEventListener = new MeetingEventListener() {
@Override
public void onMeetingJoined() {
Log.d("#meeting", "onMeetingJoined()");
}
@Override
public void onParticipantJoined(Participant participant) {
Log.d("#meeting", participant.getDisplayName() + " left");
}
}
Got a Question? Ask us on discord