Interactive Livestream (HLS) - Android
Interactive live streaming (HLS) refers to a type of live streaming where viewers can actively engage with the content being streamed and with other viewers in real-time.
In an interactive live stream (HLS), viewers can take part in a variety of activities like live polling, Q&A sessions, and even sending virtual gifts to the content creator or each other.
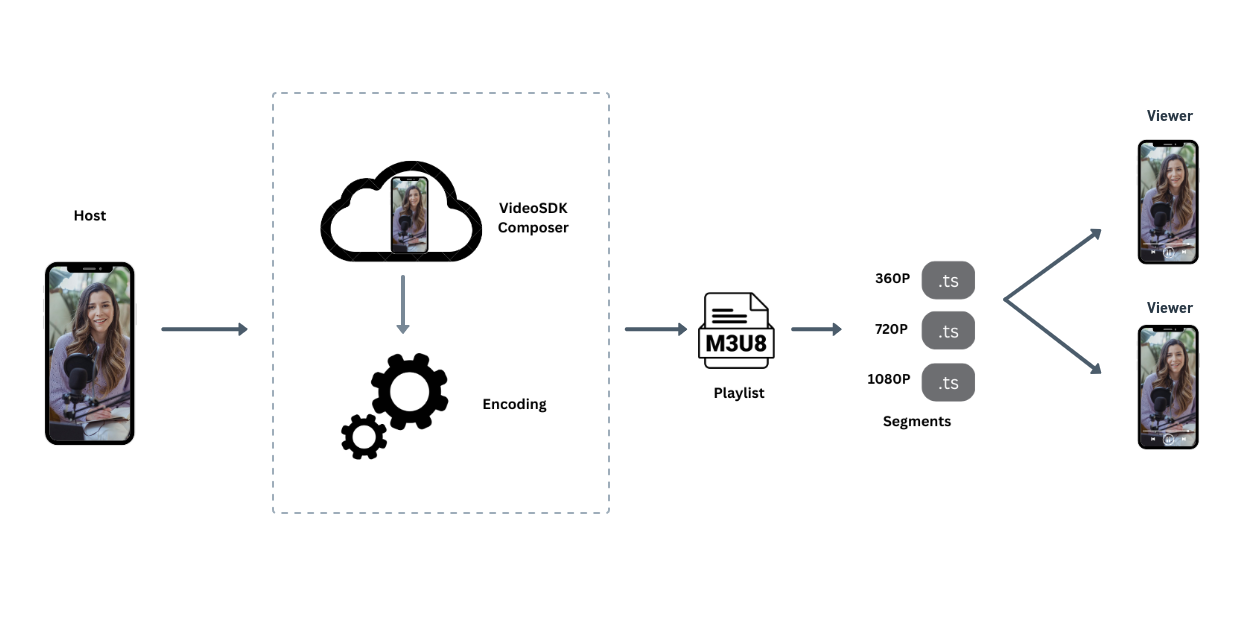
VideoSDK also allows you to configure the interactive livestream layouts in numerous ways like by simply setting different prebuilt layouts in the configuration or by providing your own custom template to do the livestream according to your layout choice.
This guide will provide an overview of how to implement start and stop Interactive live streaming (HLS).
To initiate automatic Interactive live streaming (HLS) at the beginning of a session
, simply provide the autoStartConfig
feature hls
during room
creation. For more information on configuring the autoStartConfig
, please refer to the provided documentation here.
startHls()
​
startHls()
can be used to start a interactive livestream of the meeting which can be accessed from the Meeting
class. This method accepts two parameter:
-
config
: This parameter will define how the interactive livestream layout should look like. -
transcription
: This parameter lets you start post transcription for the Livestreaming.
- Kotlin
- Java
val config = JSONObject()
// Layout Configuration
val layout = JSONObject()
JsonUtils.jsonPut(layout, "type", "GRID") // "SPOTLIGHT" | "SIDEBAR", Default : "GRID"
JsonUtils.jsonPut(layout, "priority", "SPEAKER") // "PIN", Default : "SPEAKER"
JsonUtils.jsonPut(layout, "gridSize", 4) // MAX : 25
JsonUtils.jsonPut(config, "layout", layout)
// Theme of interactive livestream layout
JsonUtils.jsonPut(config, "theme", "DARK") // "LIGHT" | "DEFAULT"
// `mode` is used to either interactive livestream video & audio both or only audio.
JsonUtils.jsonPut(config, "mode", "video-and-audio") // "audio", Default : "video-and-audio"
// Quality of interactive livestream and is only applicable to `video-and-audio` type mode.
JsonUtils.jsonPut(config, "quality", "high") // "low" | "med", Default : "med"
// This mode refers to orientation of interactive livestream.
// landscape : Start interactive livestream of the meeting in horizontally
// portrait : Start interactive livestream of the meeting in vertically (Best for mobile view)
JsonUtils.jsonPut(config, "orientation", "portrait") // "landscape", Default : "landscape"
// Post Transcription Configuration
val prompt = "Write summary in sections like Title, Agenda, Speakers, Action Items, Outlines, Notes and Summary"
val summaryConfig = SummaryConfig(true, prompt)
val modelId = "raman_v1"
val transcription = PostTranscriptionConfig(true, summaryConfig, modelId)
meeting.startHls(config, transcription)
JSONObject config = new JSONObject();
// Layout Configuration
JSONObject layout = new JSONObject();
JsonUtils.jsonPut(layout, "type", "GRID"); // "SPOTLIGHT" | "SIDEBAR", Default : "GRID"
JsonUtils.jsonPut(layout, "priority", "SPEAKER"); // "PIN", Default : "SPEAKER"
JsonUtils.jsonPut(layout, "gridSize", 4); // MAX : 25
JsonUtils.jsonPut(config, "layout", layout);
// Theme of interactive livestream layout
JsonUtils.jsonPut(config, "theme", "DARK"); // "LIGHT" | "DEFAULT"
// `mode` is used to either interactive livestream video & audio both or only audio.
JsonUtils.jsonPut(config, "mode", "video-and-audio"); // "audio", Default : "video-and-audio"
// Quality of interactive livestream and is only applicable to `video-and-audio` type mode.
JsonUtils.jsonPut(config, "quality", "high"); // "low" | "med", Default : "med"
// This mode refers to orientation of interactive livestream.
// landscape : Start interactive livestream of the meeting in horizontally
// portrait : Start interactive livestream of the meeting in vertically (Best for mobile view)
JsonUtils.jsonPut(config, "orientation", "portrait"); // "landscape", Default : "landscape"
// Post Transcription Configuration
String prompt = "Write summary in sections like Title, Agenda, Speakers, Action Items, Outlines, Notes and Summary";
SummaryConfig summaryConfig = new SummaryConfig(true, prompt);
String modelId = "raman_v1";
PostTranscriptionConfig transcription = new PostTranscriptionConfig(true, summaryConfig, modelId);
meeting.startHls(config, transcription);
stopHls()
​
stopHls()
is used to stop interactive livestream of the meeting which can be accessed from theMeeting
class.
Example​
- Kotlin
- Java
// keep track of hls
val hls = false
findViewById<View>(R.id.btnHls).setOnClickListener { view: View? ->
if (!hls) {
val config = JSONObject()
val layout = JSONObject()
JsonUtils.jsonPut(layout, "type", "GRID")
JsonUtils.jsonPut(layout, "priority", "SPEAKER")
JsonUtils.jsonPut(layout, "gridSize", 4)
JsonUtils.jsonPut(config, "layout", layout)
JsonUtils.jsonPut(config, "theme", "DARK")
JsonUtils.jsonPut(config, "mode", "video-and-audio")
JsonUtils.jsonPut(config, "quality", "high")
JsonUtils.jsonPut(config, "orientation", "portrait")
// Post Transcription Configuration
val prompt = "Write summary in sections like Title, Agenda, Speakers, Action Items, Outlines, Notes and Summary"
val summaryConfig = SummaryConfig(true, prompt)
val modelId = "raman_v1"
val transcription = PostTranscriptionConfig(true, summaryConfig, modelId)
// Start Hls
meeting!!.startHls(config)
} else {
// Stop Hls
meeting!!.stopHls()
}
}
// keep track of hls
boolean hls = false;
findViewById(R.id.btnHls).setOnClickListener(view -> {
if (!hls) {
JSONObject config = new JSONObject();
JSONObject layout = new JSONObject();
JsonUtils.jsonPut(layout, "type", "GRID");
JsonUtils.jsonPut(layout, "priority", "SPEAKER");
JsonUtils.jsonPut(layout, "gridSize", 4);
JsonUtils.jsonPut(config, "layout", layout);
JsonUtils.jsonPut(config, "theme", "DARK");
JsonUtils.jsonPut(config, "mode", "video-and-audio");
JsonUtils.jsonPut(config, "quality", "high");
JsonUtils.jsonPut(config, "orientation", "portrait");
// Post Transcription Configuration
String prompt = "Write summary in sections like Title, Agenda, Speakers, Action Items, Outlines, Notes and Summary";
SummaryConfig summaryConfig = new SummaryConfig(true, prompt);
String modelId = "raman_v1";
PostTranscriptionConfig transcription = new PostTranscriptionConfig(true, summaryConfig, modelId);
// Start Hls
meeting.startHls(config);
} else {
// Stop Hls
meeting.stopHls();
}
});
If you want to learn more about the Interactive Livestream and how you can implement it in your own platform, you can checkout this guide.
Event associated with HLS​
-
onHlsStateChanged - Whenever meeting HLS state changes, then
onHlsStateChanged
event will trigger. -
You can get the
playbackHlsUrl
andlivestreamUrl
of the HLS to play it on the Viewer side when the state changes toHLS_PLAYABLE
.playbackHlsUrl
- Live HLS with playback supportlivestreamUrl
- Live HLS without playback support
downstreamUrl
is now depecated. Use playbackHlsUrl
or livestreamUrl
in place of downstreamUrl
- Kotlin
- Java
private val meetingEventListener: MeetingEventListener = object : MeetingEventListener() {
override fun onHlsStateChanged(HlsState: JSONObject) {
when (HlsState.getString("status")) {
"HLS_STARTING" -> Log.d("onHlsStateChanged", "Meeting hls is starting")
"HLS_STARTED" -> Log.d("onHlsStateChanged", "Meeting hls is started")
"HLS_PLAYABLE" -> {
Log.d("onHlsStateChanged", "Meeting hls is playable now")
// on hls playable you will receive playbackHlsUrl and livestreamUrl
val playbackHlsUrl = HlsState.getString("playbackHlsUrl")
val livestreamUrl = HlsState.getString("livestreamUrl")
}
"HLS_STOPPING" -> Log.d("onHlsStateChanged", "Meeting hls is stopping")
"HLS_STOPPED" -> Log.d("onHlsStateChanged", "Meeting hls is stopped")
}
}
}
override fun onCreate(savedInstanceState: Bundle?) {
//...
// add listener to meeting
meeting!!.addEventListener(meetingEventListener)
}
private final MeetingEventListener meetingEventListener = new MeetingEventListener() {
@Override
public void onHlsStateChanged(JSONObject HlsState) {
switch (HlsState.getString("status")) {
case "HLS_STARTING":
Log.d("onHlsStateChanged", "Meeting hls is starting");
break;
case "HLS_STARTED":
Log.d("onHlsStateChanged", "Meeting hls is started");
break;
case "HLS_PLAYABLE":
Log.d("onHlsStateChanged", "Meeting hls is playable now");
// on hls started you will receive playbackHlsUrl and livestreamUrl
String playbackHlsUrl = HlsState.getString("playbackHlsUrl");
String livestreamUrl = HlsState.getString("livestreamUrl");
break;
case "HLS_STOPPING":
Log.d("onHlsStateChanged", "Meeting hls is stopping");
break;
case "HLS_STOPPED":
Log.d("onHlsStateChanged", "Meeting hls is stopped");
break;
}
}
}
@Override
protected void onCreate(Bundle savedInstanceState) {
//...
// add listener to meeting
meeting.addEventListener(meetingEventListener);
}
Custom Template​
With VideoSDK, you can also use your own custom designed layout template to livestream the meetings. In order to use the custom template, you need to create a template for which you can follow this guide. Once you have setup the template, you can use the REST API to start the livestream with the templateURL
parameter.
API Reference​
The API references for all the methods utilised in this guide are provided below.
Got a Question? Ask us on discord