What is SurfaceViewRender ?
SurfaceViewRender
is a very powerful component of WebRTC
since it is used to display the video stream on a SurfaceView. You can use SurfaceViewRender
to show video of participant in your screen.
Here's the lifecycle of a SurfaceViewRenderer:
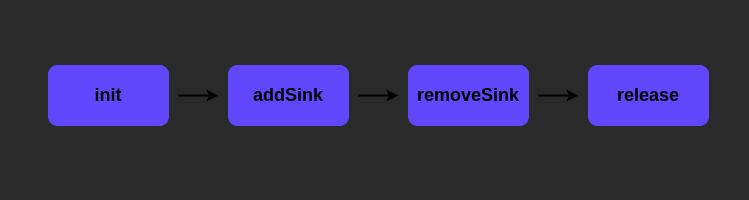
Which means:
-
The SurfaceViewRenderer must be first initialised after being created and before it can be use at all. It starts a render thread.
-
addSink()
needs to be called when you are having VideoTrack to render. addSink is method ofVideoTrack
that you want to add to it. You have to passsurfaceViewRender
as parameter. -
removeSink()
should only be called when you're done showing the video. Another method ofVideoTrack
, and it takes thesurfaceViewRenderer
as a parameter. -
Release at last to allow the render thread to terminate. This function should be called before the activity is destroyed and/or before init it again.
Mistakes you might make and stuck :​
1. Not initialised surfaceViewRender​
The video never appears since a render thread was never start and you will see black view.
2. Init it again before releasing it.​
If you are not releasing surfaceViewRender before calling init()
again, you will get this error.
java.lang.IllegalStateException: svrParticipant Already initialized
at org.webrtc.EglRenderer.init(EglRenderer.java:212)
3. Two videos render on same SurfaceViewRender​
If you called addSink()
on the same SurfaceViewRenderer
for two different tracks and did not call removeSink
before that, then you will see two different videos on same SurfaceViewRenderer with flickers which would look like this :
Too complicated right? However, VideoSDK makes it simple for you. You can use VideoView
instead of SurfaceViewRenderer
.
- If you want to use
VideoView
, please update SDK version to0.1.13
or higher.
VideoView​
VideoView
provides a better abstraction to render live video and handles edge cases like managing init state, render more than one videos on same surfaceViewRender.
Methods​
-
addTrack(VideoTrack videoTrack) -
addTrack
will initialised SurfaceViewRender for you and render videoTrack that you provided. -
removeTrack() -
removeTrack
should only be called when you're done showing the video. -
releaseSurfaceViewRenderer() -
releaseSurfaceViewRenderer
should be called before the activity is destroyed.
How to use VideoView ?​
Layout​
// <org.webrtc.SurfaceViewRenderer
// android:id="@+id/svrParticipant"
// android:layout_width="match_parent"
// android:layout_height="match_parent" />
<live.videosdk.rtc.android.VideoView
android:id="@+id/participantView"
android:layout_width="match_parent"
android:layout_height="match_parent"
/>
Initalising and adding video track​
- Kotlin
- Java
// svrParticipant.init(PeerConnectionUtils.getEglContext(), null)
// var track = stream.getTrack() as VideoTrack
// track!!.addSink(svrParticipant)
val track = stream.track as VideoTrack
participantView!!.addTrack(track);
// svrParticipant.init(PeerConnectionUtils.getEglContext(), null);
// VideoTrack track = (VideoTrack) stream.getTrack();
// track.addSink(svrParticipant);
VideoTrack track = (VideoTrack) stream.getTrack();
participantView.addTrack(track);
removeTrack​
- Kotlin
- Java
// var track = stream.track as VideoTrack
// track!!.removeSink(svrParticipant);
participantView.removeTrack();
// VideoTrack track = (VideoTrack) stream.getTrack();
// if (track != null) track.removeSink(svrParticipant);
participantView.removeTrack();
release​
- Kotlin
- Java
override fun onDestroy() {
participantView.releaseSurfaceViewRenderer()
super.onDestroy()
}
@Override
protected void onDestroy() {
participantView.releaseSurfaceViewRenderer();
super.onDestroy();
}
Got a Question? Ask us on discord