Optimize Video Tracks - Android
While optimizing for the best viewing experience, it is necessary to fine-tune the video tracks that are being used during the calls.
For the best fine-tuning experience, we have introduced the ability to pass a custom video track for the participant's media before and during the meeting.
Custom Video Track​
This feature can be used to add custom video encoder configurations, optimization mode (whether you want to focus on motion, text or detail of the video) and background removal & video filter from external SDK(e.g., Banuba)and send it to other participants.
How to Create a Custom Video Track ?
​
- You can create a Video Track using
createCameraVideoTrack()
method ofVideoSDK
. - This method can be used to create video track using different encoding parameters, camera facing mode, and optimization mode and return
CustomStreamTrack
.
Example​
- Kotlin
- Java
val videoCustomTrack: CustomStreamTrack = VideoSDK.createCameraVideoTrack(
// This will accept the resolution (height x width) of video you want to capture.
"h720p_w960p", // "h720p_w960p" | "h720p_w1280p" ... // Default : "h480p_w720p"
// It will specify whether to use front or back camera for the video track.
"front", "back", Default : "front"
// We will discuss this parameter in next step.
CustomStreamTrack.VideoMode.MOTION, // CustomStreamTrack.VideoMode.TEXT, CustomStreamTrack.VideoMode.DETAIL , Default : CustomStreamTrack.VideoMode.MOTION
// multiStream - we will discuss this parameter in next step.
false, // true
// Pass Context
this,
// This is Optional parameter. We will discuss this parameter in next step.
observer)
CustomStreamTrack customStreamTrack = VideoSDK.createCameraVideoTrack(
// This will accept the resolution (height x width) of video you want to capture.
"h480p_w640p", // "h720p_w960p" | "h720p_w1280p" ... // Default : "h480p_w640p"
// It will specify whether to use front or back camera for the video track.
"front", // "back, Default : "front""
// We will discuss this parameter in next step.
CustomStreamTrack.VideoMode.MOTION, // CustomStreamTrack.VideoMode.TEXT, CustomStreamTrack.VideoMode.DETAIL , Default : CustomStreamTrack.VideoMode.MOTION
// multiStream - we will discuss this parameter in next step.
false, // true
// Pass Context
this
// This is Optional parameter. We will discuss this parameter in next step.
observer);
The capabilities of the device have a significant impact on how custom track configurations behave. Assuming a case where you set encoder configuration to 1080p but the camera only supports 720p, then encoder configuration will automatically switch to the highest resolution that the device can handle, which is 720p.
What is optimizationMode
?​
-
It will specify the optimization mode for the video track being generated.
-
motion
: This type of track should more focus on motion video. For example, webcam video, movies or video games.- This type of track will degrade
resolution
in order to maintainframe rate
.
- This type of track will degrade
-
text
: This type of track should more focus on significant sharp edges and areas of consistent color that can change frequently. For example, presentations or web pages with text content.- This type of track will degrade
frame rate
in order to maintainresolution
.
- This type of track will degrade
-
detail
: This type of track should more focus on details of the video. For example, presentations, painting or line art.- This type of track will degrade
frame rate
in order to maintainresolution
.
- This type of track will degrade
What is multiStream
?​
- It will specify if the stream should send multiple resolution layers or single resolution layer.
multiStream : true
By default, VideoSDK sends multiple resolution video streams to the server (whether you are using custom video track or not), For instance, user device capabilty is 720p, so VideoSDK sends 720p along with 640p and 480p streams. This allows VideoSDK to deliver the appropriate stream to each participant based on their network bandwidth.
multiStream : false
If you want to restrict the VideoSDK to send only one stream to maintain quality, you can set multiStream
to false
.
setQuality
would not have any effect if multiStream is set to false
.
What is observer
?​
- If you want to use video filter from external SDK(e.g., Banuba) then pass instance of
CapturerObserver
in this parameter.
For Banuba integraion with VideoSDK, please visit Banuba Intergation with VideoSDK
How to Setup a Custom Video Track ?
​
The custom track can be set up both before and after the initialization of the meeting.
- Setting up a Custom Track during the initialization of a meeting
- Setting up a Custom Track with methods
1. Setting up a Custom Track during the initialization of a meeting​
If you are passing webcamEnabled: true
in the initMeeting
of VideoSDK
and want to use custom tracks from start of the meeting, you can pass custom track in the initMeeting
as shown below.
Custom Track will not apply on webcamEnabled: false
configuration.
Example​
- Kotlin
- Java
override fun onCreate(savedInstanceState: Bundle?) {
//..
val customTracks: MutableMap<String, CustomStreamTrack> = HashMap()
val videoCustomTrack: CustomStreamTrack =
VideoSDK.createCameraVideoTrack("h720p_w960p", "front", CustomStreamTrack.VideoMode.MOTION, false, this)
customTracks["video"] = videoCustomTrack //Key must be "video"
// create a new meeting instance
val meeting = VideoSDK.initMeeting(
this@MainActivity, meetingId, participantName,
//MicEnabled
true,
//WebcamEnabled , If true, it will use the passed custom track to turn webcam on
true,
// ParticipantId
null,
// Mode
null,
// MultiStream
false,
//Pass the custom tracks here
customTracks,
//MetaData
null
)
}
@Override
protected void onCreate(Bundle savedInstanceState) {
//..
Map<String, CustomStreamTrack> customTracks = new HashMap<>();
CustomStreamTrack videoCustomTrack = VideoSDK.createCameraVideoTrack("h720p_w960p", "front", CustomStreamTrack.VideoMode.MOTION, false, this);
customTracks.put("video", videoCustomTrack); //Key must be "video"
// create a new meeting instance
Meeting meeting = VideoSDK.initMeeting(
MainActivity.this, meetingId, participantName,
//MicEnabled
true,
//WebcamEnabled , If true, it will use the passed custom track to turn webcam on
true,
// ParticipantId
null,
// Mode
null,
// MultiStream
false,
//Pass the custom tracks here
customTracks,
//MetaData
null
);
}
2. Setting up a Custom Track with methods​
In order to switch tracks during the meeting, you have to pass the CustomStreamTrack
in the enableWebcam()
method of Meeting
.
Make sure to call disableWebcam()
before you create a new track as it may lead to unexpected behavior.
Example​
- Kotlin
- Java
val customStreamTrack: CustomStreamTrack = VideoSDK.createCameraVideoTrack("h720p_w960p", "back", CustomStreamTrack.VideoMode.MOTION, false, this)
meeting!!.enableWebcam(customStreamTrack)
CustomStreamTrack customStreamTrack=VideoSDK.createCameraVideoTrack("h720p_w960p", "back", CustomStreamTrack.VideoMode.MOTION, false, this);
meeting.enableWebcam(customStreamTrack);
Which Configuration is suitable for Device ?
​
In this section, we will understand participant size wise encoder(Resolution)
and multiStream
configuration.
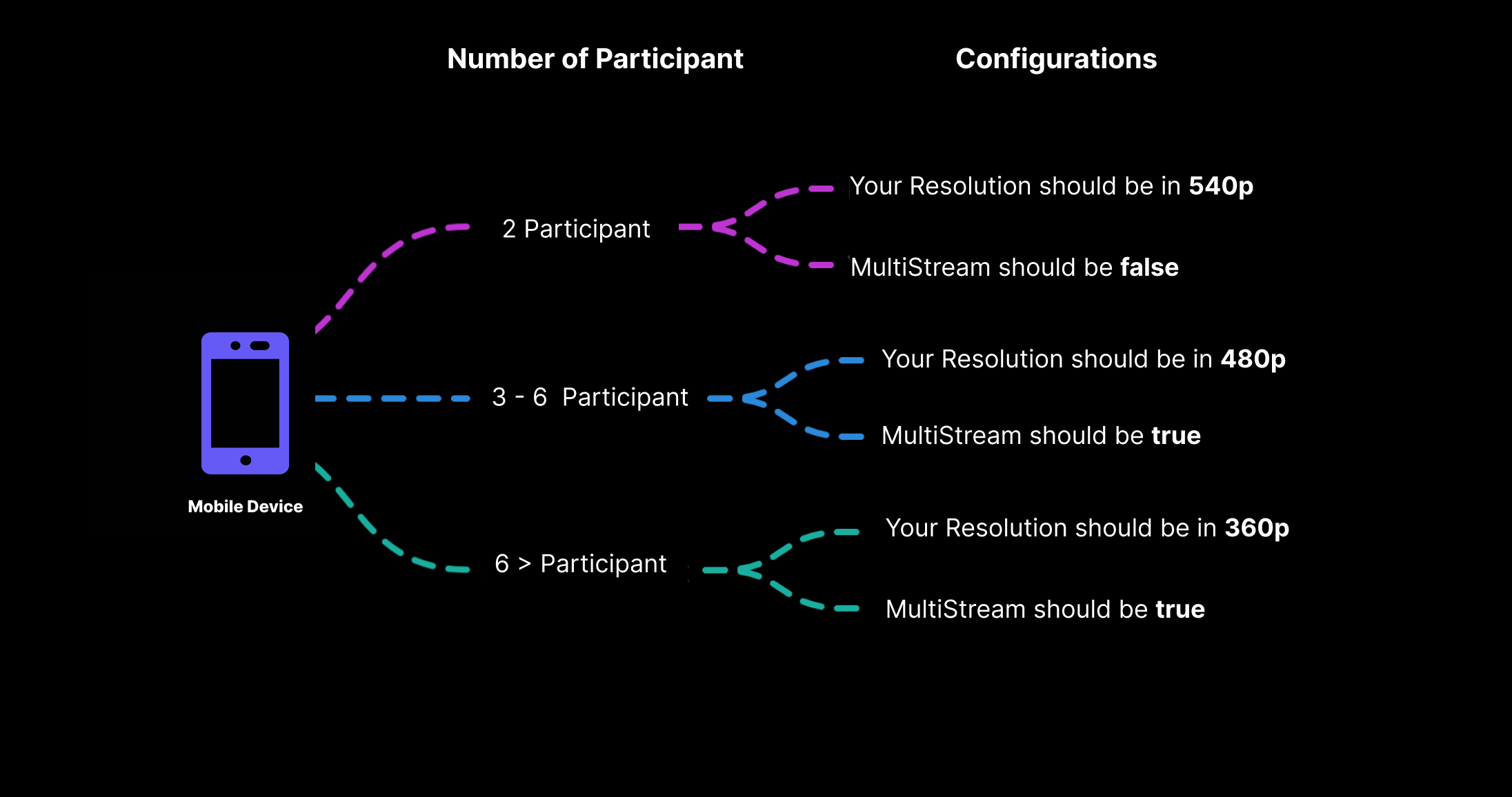
Custom Screen Share Track​
This feature can be used to customise screenshare streams with enhanced optimization mode and predefined encoderConfig(Resolution + FPS) for specific use-case and send it to other participants.
How to Create Custom Screen Share Track ?
​
- You can create a Screen Share track using
createScreenShareVideoTrack()
method ofVideoSDK
. - This method can be used to create video track using different encoding parameters and optimization mode.
Example​
- Kotlin
- Java
// data is received from onActivityResult method.
VideoSDK.createScreenShareVideoTrack(
// This will accept the height & FPS of video you want to capture.
"h720p_15fps", // `h360p_30fps` | `h1080p_30fps` // Default : `h720p_15fps`
// It is Intent received from onActivityResult when user provide permission for ScreenShare.
data,
// Pass Conext
this)
//Callback to this listener will be made when track is ready with CustomTrack as parameter
{
track ->
meeting!!.enableScreenShare(track)
}
// data is received from onActivityResult method.
VideoSDK.createScreenShareVideoTrack(
// This will accept the height & FPS of video you want to capture.
"h720p_15fps", // `h360p_30fps` | `h1080p_30fps` // Default : `h720p_15fps`
/highlight-next-line
// It is Intent received from onActivityResult when user provide permission for ScreenShare
data,
// Pass Conext
this,
//Callback to this listener will be made when track is ready with CustomTrack as parameter
(track)->{meeting.enableScreenShare(track);}
);
How to Setup Custom Screen Share Track ?
​
In order to switch tracks during the meeting, you have to pass the CustomStreamTrack
in the enableScreenShare()
method of Meeting
.
Make sure to call disableScreenShare()
before you create a new track as it may lead to unexpected behavior.
Example​
- Kotlin
- Java
@TargetApi(21)
private fun askPermissionForScreenShare() {
val mediaProjectionManager = application.getSystemService<Any>(
Context.MEDIA_PROJECTION_SERVICE
) as MediaProjectionManager
startActivityForResult(
mediaProjectionManager.createScreenCaptureIntent(), CAPTURE_PERMISSION_REQUEST_CODE
)
}
@RequiresApi(api = Build.VERSION_CODES.LOLLIPOP)
override fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent?) {
super.onActivityResult(requestCode, resultCode, data)
if (requestCode != CAPTURE_PERMISSION_REQUEST_CODE) return
if (resultCode == RESULT_OK) {
VideoSDK.createScreenShareVideoTrack("h720p_15fps", data, this) { track ->
meeting!!.enableScreenShare(track)
}
}
}
@TargetApi(21)
private void askPermissionForScreenShare() {
MediaProjectionManager mediaProjectionManager =
(MediaProjectionManager) getApplication().getSystemService(
Context.MEDIA_PROJECTION_SERVICE);
startActivityForResult(
mediaProjectionManager.createScreenCaptureIntent(), CAPTURE_PERMISSION_REQUEST_CODE);
}
@RequiresApi(api = Build.VERSION_CODES.LOLLIPOP)
@Override
public void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode != CAPTURE_PERMISSION_REQUEST_CODE)
return;
if (resultCode == Activity.RESULT_OK) {
VideoSDK.createScreenShareVideoTrack("h720p_15fps", data, this, (track)->{
meeting.enableScreenShare(track);
});
}
}
API Reference​
The API references for all the methods and events utilised in this guide are provided below.
Got a Question? Ask us on discord