Mute / Unmute Mic - Flutter
This feature allows hosts to communicate with each other during the livestream by enabling or disabling their microphones. While only hosts (participants in SEND_AND_RECV mode) can speak, audience members (in RECV_ONLY mode) can listen to the conversation in real time.
unmuteMic()
​
-
By using the
unmuteMic()
function of theRoom
class, the host can publish their audio to other hosts and audience members. -
You can also pass a customised audio track in the
unmuteMic()
method by using Custom Audio Track. -
Audio stream of the participant can be accessed from the
stream
map of theParticipant
object.
muteMic()
​
- By using the
muteMic()
function of theRoom
class, the host can stop publishing their audio to other hosts and audience members.
Events associated with unmuteMic​
- Every Participant will receive a callback on
Events.streamEnabled
of theParticipant
object withStream
object.
Events associated with muteMic​
- Every Participant will receive a callback on
Events.streamDisabled
of theParticipant
object withStream
object.
import 'package:flutter/material.dart';
import 'package:videosdk/videosdk.dart';
class ParticipantTile extends StatefulWidget {
final Participant participant;
const ParticipantTile({super.key, required this.participant});
@override
State<ParticipantTile> createState() => _ParticipantTileState();
}
class _ParticipantTileState extends State<ParticipantTile> {
@override
void initState() {
// initial video stream for the participant
//Check if camera stream is already present
widget.participant.streams.forEach((key, Stream stream) {
setState(() {
if (stream.kind == 'audio') {
audioStream = stream;
}
});
});
_initStreamListeners();
super.initState();
}
//Change state according to the events received
_initStreamListeners() {
widget.participant.on(Events.streamEnabled, (Stream stream) {
if (stream.kind == 'audio') {
//Mic Turned On
setState(() => audioStream = stream);
}
});
widget.participant.on(Events.streamDisabled, (Stream stream) {
if (stream.kind == 'audio') {
//Mic Turned Off
setState(() => audioStream = null);
}
});
}
@override
Widget build(BuildContext context) {
return Column(
children:[
ElevatedButton(
onPressed:(){
_room.unmuteMic();
},
child: const Text("Unmute Mic"),
),
ElevatedButton(
onPressed:(){
_room.muteMic();
},
child: const Text("Mute Mic"),
),
]
);
}
}
Audio Permissions​
- If permissions are not granted, use the
requestPermission()
method of theVideoSDK
class to prompt users to grant access to their devices. - To enable requesting of microphone and camera permissions on iOS devices, add the following to your
Podfile
:
post_install do |installer|
installer.pods_project.targets.each do |target|
flutter_additional_ios_build_settings(target)
target.build_configurations.each do |config|
//Add this into your podfile
config.build_settings['GCC_PREPROCESSOR_DEFINITIONS'] ||= [
'PERMISSION_MICROPHONE=1',
]
end
end
end
In case permissions are blocked by the user, the permission request dialogue cannot be re-rendered programmatically. In such cases, consider providing guidance to users on manually adjusting their permissions.
void requestMediaPermissions() async {
try {
//For requesting just audio permission.
Map<String, bool>? reqAudioPermissions = await VideoSDK.requestPermissions(Permissions.audio);
;
} catch (ex) {
print("Error in requestPermission ");
}
};
- The
requestPermissions()
method is not supported on Desktop Applications and Firefox Browser.
- Requesting permissions if not already granted:
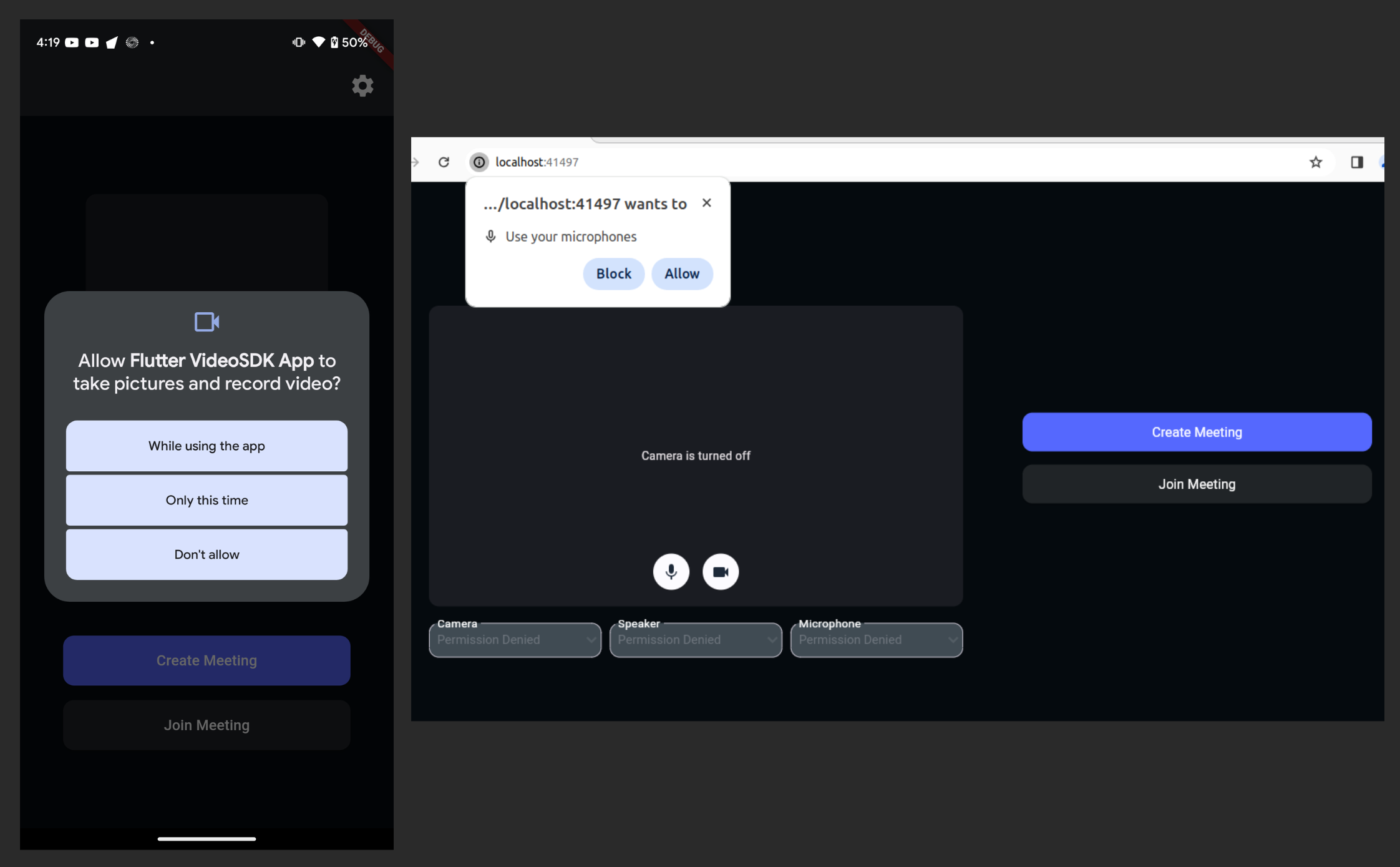
API Reference​
The API references for all the methods and events utilised in this guide are provided below.
Got a Question? Ask us on discord