On / Off Camera - Flutter
This feature enables hosts to turn their cameras on or off to share their video stream with other hosts and audience members during the livestream. Only hosts (in SEND_AND_RECV
mode) can broadcast their camera feed, while audience members (in RECV_ONLY
mode) can view it in real time.
enableCam()
-
By using the
enableCam()
function of theRoom
class, the host can publish their video to to other hosts and audience members. -
You can also pass a customised video track in
enableCam()
by using Custom Video Track. -
Video stream of the participant can be accessed from the
streams
property ofParticipant
object.
disableCam()
- By using the
disableCam()
function of theRoom
Class, the host can stop publishing their video to to other hosts and audience members.
Events associated with enableCam
- Every Participant will receive a callback on
Events.streamEnabled
of theParticipant
object withStream
object.
Events associated with disableCam
- Every Participant will receive a callback on
Events.streamDisabled
of theParticipant
object withStream
object.
import 'package:flutter/material.dart';
import 'package:videosdk/videosdk.dart';
class LiveStreamScreen extends StatefulWidget {
...
}
class _LiveStreamScreenState extends State<LiveStreamScreen> {
late Room _room;
@override
void initState() {
// initial video stream for the participant
//Check if camera stream is already present
widget.participant.streams.forEach((key, Stream stream) {
setState(() {
if (stream.kind == 'video') {
videoStream = stream;
}
});
});
_initStreamListeners();
}
@override
Widget build(BuildContext context) {
return Column(
children:[
ElevatedButton(
onPressed:(){
_room.enableCam();
},
child: const Text("Enable Camera"),
),
ElevatedButton(
onPressed:(){
_room.disableCam();
},
child: const Text("Disable Camera"),
),
]
);
}
//Change state according to the events received
_initStreamListeners() {
widget.participant.on(Events.streamEnabled, (Stream stream) {
if (stream.kind == 'video') {
setState(() => videoStream = stream);
}
});
widget.participant.on(Events.streamDisabled, (Stream stream) {
if (stream.kind == 'video') {
setState(() => videoStream = null);
}
});
}
}
Video Permissions
- If permissions are not granted, use the
requestPermission()
method of theVideoSDK
class to prompt users to grant access to their devices. - To enable requesting of microphone and camera permissions on iOS devices, add the following to your
Podfile
:
post_install do |installer|
installer.pods_project.targets.each do |target|
flutter_additional_ios_build_settings(target)
target.build_configurations.each do |config|
//Add this into your podfile
config.build_settings['GCC_PREPROCESSOR_DEFINITIONS'] ||= [
'PERMISSION_CAMERA=1',
]
end
end
end
In case permissions are blocked by the user, the permission request dialogue cannot be re-rendered programmatically. In such cases, consider providing guidance to users on manually adjusting their permissions.
void requestMediaPermissions() async {
try {
//For requesting just video permission.
Map<String, bool>? reqVideoPermissions = await VideoSDK.requestPermissions(Permissions.video);
} catch (ex) {
print("Error in requestPermission ");
}
};
- The
requestPermissions()
method is not supported on Desktop Applications and Firefox Browser.
- Requesting permissions if not already granted:
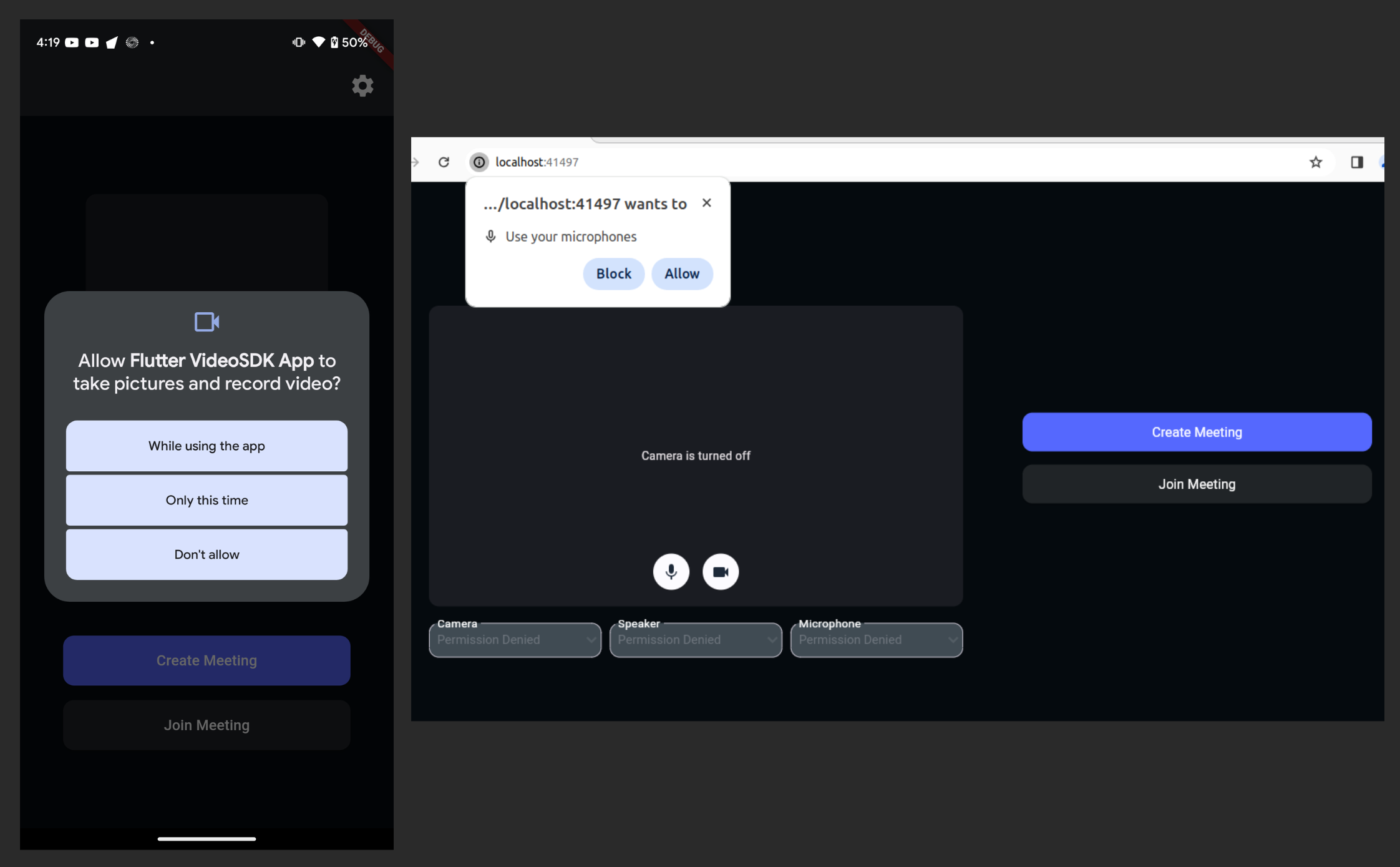
API Reference
The API references for all the methods and events utilised in this guide are provided below.
Got a Question? Ask us on discord