Virtual Background(BETA) - Flutter
Virtual backgrounds let you replace your real background during video calls or meetings with a digital image or video. This can be useful for:
-
Privacy: Hide your messy room or anything you don't want others to see.
-
Focus: Minimize distractions and keep the focus on the conversation.
-
Fun: Liven up the call with a cool background theme.
Virtual background is currently in BETA and may not perform optimally on certain devices or with custom tracks that have high resolutions.
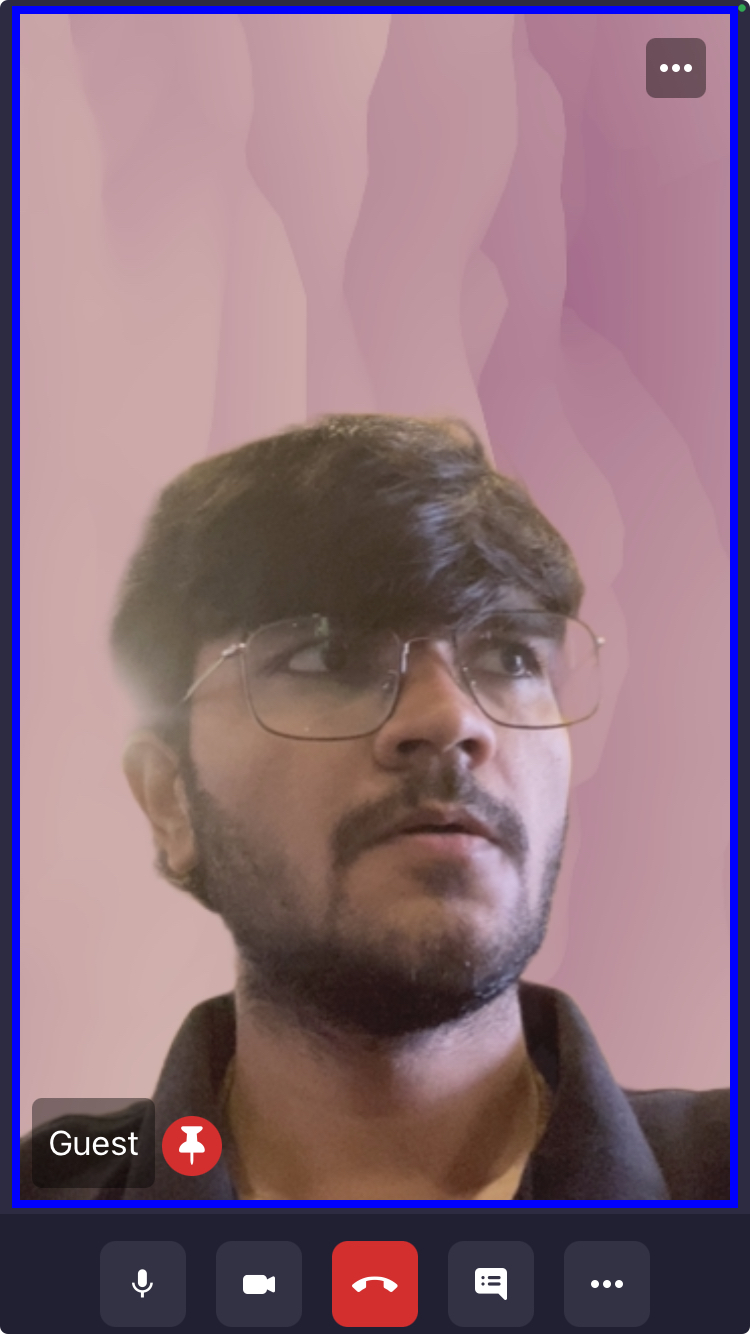
Virtual Background using VideoSDKMediaEffects
plugin​
The VideoSDKMediaEffects
plugin enables the integration of sophisticated virtual background effects within your video applications. By leveraging this plugin, users can replace their physical backgrounds with custom images or dynamic video backdrops, creating a more immersive and engaging experience.
- The Virtual Background methods from the
VideoSDKMediaEffects
plugin are supported only on Android and iOS devices. - The Virtual Background feature in VideoSDK can be utilized regardless of the meeting environment, including the pre-call screen.
Install the plugin​
- Install the
VideoSDKMediaEffects
plugin using the below-mentioned flutter command. Make sure you are in your flutter app directory before you run this command.
$ flutter pub add videosdk_media_effects
Apply Virtual Background​
- The
applyVirtualBackground()
method from theVideoSDKMediaEffects
plugin allows users to dynamically change the video background during a live session. By passing a URI of the desired background image, this method seamlessly integrates the virtual background into the video stream.
Change Virtual Background​
- The
changeVirtualBackground()
method from theVideoSDKMediaEffects
plugin enables users to effortlessly switch their current virtual background to a new image. This functionality allows for dynamic customization and enhances the user's video experience by providing the ability to update their background on the fly. By passing a URI of the new background image, this method seamlessly integrates the new virtual background into the video stream.
Remove Virtual Background​
- The
removeVirtualBackground()
method from theVideoSDKMediaEffects
plugin provides users with a convenient way to revert their video background to its original state, removing any previously applied virtual background. This functionality ensures flexibility for users to switch between virtual and real backgrounds as needed.
Example Code:
import 'package:flutter/material.dart';
import 'package:videosdk/videosdk.dart';
//Import the plugin
import 'package:videosdk_media_effects/videosdk_media_effects.dart';
class MeetingScreen extends StatefulWidget {
//Existing configuration
}
class _MeetingScreenState extends State<MeetingScreen> {
late Room _room;
@override
void initState() {
//Existing configuration
}
@override
Widget build(BuildContext context) {
return Column(
children:[
//To Apply Virtual Background
ElevatedButton(
onPressed:(){
Uri backgroundImageURI = Uri.parse("https://cdn.videosdk.live/virtual-background/cloud.jpeg");
VideosdkMediaEffects.applyVirtualBackground(backgroundSource: backgroundImageURI);
},
child: const Text("Apply Virtual Background"),
),
//To Change Virtual Background
ElevatedButton(
onPressed:(){
Uri newBackgroundImageURI = Uri.parse("https://cdn.videosdk.live/virtual-background/san-fran.jpeg");
VideosdkMediaEffects.changeVirtualBackground(backgroundSource: newBackgroundImageURI);
},
child: const Text("Change Virtual Background"),
),
//To Remove Virtual Background
ElevatedButton(
onPressed:(){
VideosdkMediaEffects.removeVirtualBackground();
},
child: const Text("Remove Virtual Background"),
),
]
);
}
}
API Reference​
The API references for all the methods utilized in this guide are provided below.
Got a Question? Ask us on discord