Start or Join Meeting - iOS
After the successful installation of VideoSDK, the next step is to integrate VideoSDK features with your webApp/MobileApp.
To Communicate with other participant's audio or video call, you will need to join the meeting.
This guide will provide an overview of how to configure, initialize and join a VideoSDK meeting.
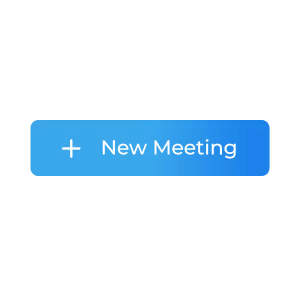
1. Configuration​
To configure a meeting, you will need generated token and meetingId, we had discussed in Server Setup. This code snippet calls API from local server
Scenario 1 - Suppose you don't have any meetingId, you can simply generate meetingId by invoking create-meeting
API.
Scenario 2 - Suppose you have meetingId, now you don't have to call create-meeting
API to generate meetingId, instead you can call validate-meeting
API to validate meetingId.
Token generation API is necessary for both scenario.
// Update server url here.
let LOCAL_SERVER_URL = "http://192.168.0.101:9000"
class APIService {
class func getToken(completion: @escaping (Result<String, Error>) -> Void) {
var url = URL(string: LOCAL_SERVER_URL)!
url = url.appendingPathComponent("get-token")
URLSession.shared.dataTask(with: url) { data, response, error in
if let data = data, let token = data.toJSON()["token"] as? String {
completion(.success(token))
} else if let err = error {
completion(.failure(err))
}
}
.resume()
}
class func createMeeting(token: String, completion: @escaping (Result<String, Error>) -> Void) {
var url = URL(string: LOCAL_SERVER_URL)!
url = url.appendingPathComponent("create-meeting")
let params = ["token": token]
var request = URLRequest(url: url)
request.httpMethod = "POST"
request.httpBody = try? JSONSerialization.data(withJSONObject: params, options: [])
URLSession.shared.dataTask(with: request) { data, response, error in
if let data = data, let meetingId = data.toJSON()["meetingId"] as? String {
completion(.success(meetingId))
} else if let err = error {
completion(.failure(err))
}
}
.resume()
}
}
2. Initialization​
After configuration, you will have to Initialize
meeting by providing name, meetingId, micEnabled, webcamEnabled & maxResolution.
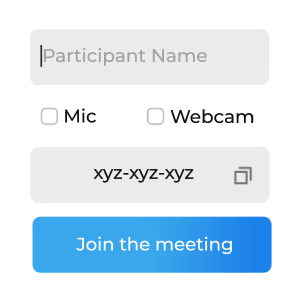
// import sdk
import VideoSDKRTC
class MeetingViewController: UIViewController {
// meeting
private var meeting: Meeting?
override func viewDidLoad() {
super.viewDidLoad()
// Configure authentication token got earlier
VideoSDK.config(token: <Authentication-token>)
// create a new meeting instance
meeting = VideoSDK.initMeeting(
meetingId: <meetingId>, // required
participantName: <participantName>, // required
micEnabled: <flag-to-enable-mic>, // optional, default: true
webcamEnabled: <flag-to-enalbe-camera> // optional, default: true
)
}
}
3. Join​
After configuration & initialization, the third step is to call join() to join a meeting.
After joining, you will be able to Manage Participant in a meeting.
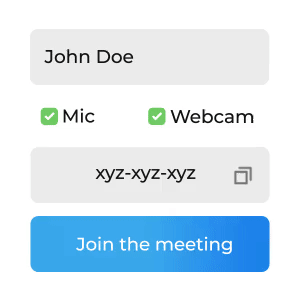
// join
meeting?.join();
API Reference​
The API references for all the methods and events utilised in this guide are provided below.
Got a Question? Ask us on discord