Quick Start for Conference in Python
VideoSDK empowers you to seamlessly integrate the video calling feature into your Python application within minutes.
In this quickstart, you'll explore the group calling feature of VideoSDK. Follow the step-by-step guide to integrate it within your application.
Prerequisites​
Before proceeding, ensure that your development environment meets the following requirements:
- VideoSDK Developer Account (Not having one? Follow VideoSDK Dashboard)
- Basic understanding of Python
- Python installed on your device.
- Python VideoSDK
- Python asyncio (optional)
You need a VideoSDK account to generate a token. Visit the VideoSDK dashboard to generate a token.
Getting Started with the Code!​
Follow the steps to create the environment necessary to add video calls to your app. You can also find the code sample for quickstart here.
Create a New Python Project​
Create a new Python project using the below command.
mkdir videosdk-python && cd videosdk-python
Install VideoSDK​
Install the VideoSDK using the pip command below.
pip install videosdk
Project Structure​
Your project structure should look like this:
videosdk-python
├── main.py
├── meeting_events.py
├── participant_events.py
├── api.py
Step 1: Create an API Call​
Before moving on, create an API request to generate a unique meetingId. You will need an authentication token, which you can create either through the videosdk-rtc-api-server-examples or directly from the VideoSDK Dashboard for developers.
import requests
# This is the Auth token, you will use it to generate a meeting and connect to it
token = "<Your-Token>"
# API call to create a meeting
def create_meeting(token):
url = "https://api.videosdk.live/v2/rooms"
headers = {
"authorization": token,
"Content-Type": "application/json"
}
response = requests.post(url, headers=headers, json={})
response_data = response.json()
room_id = response_data.get("roomId")
return room_id
# Example usage
meeting_id = create_meeting(token)
print("Meeting ID:", meeting_id)
Step 2: Join the Meeting​
Join an existing meeting or a new meeting created above.
Add the following code to the main.py
file.
import asyncio
from videosdk import MeetingConfig, VideoSDK
VIDEOSDK_TOKEN = "<TOKEN>"
MEETING_ID = "<MEETING_ID>"
NAME = "<NAME>"
loop = asyncio.get_event_loop()
def main():
meeting_config = MeetingConfig(
meeting_id=MEETING_ID,
name=NAME,
mic_enabled=True,
webcam_enabled=True,
token=VIDEOSDK_TOKEN
)
# Initialize the meeting
meeting = VideoSDK.init_meeting(**meeting_config)
print("Joining the meeting...")
# Join the meeting
meeting.join()
print("Joined successfully")
if __name__ == '__main__':
main()
loop.run_forever()
Step 3: Listen to Meeting Events​
We can listen to meeting events as callbacks by inheriting the class MeetingEventHandler
. We will use MyMeetingEventHandler
class in step 5.
from videosdk import Participant, MeetingEventHandler
from participant_events import MyParticipantEventHandler
class MyMeetingEventHandler(MeetingEventHandler):
def __init__(self):
super().__init__()
def on_meeting_joined(self, data):
print("Meeting joined:", data)
def on_meeting_left(self, data):
print("Meeting left:", data)
def on_participant_joined(self, participant: Participant):
print("Participant joined:", participant)
participant.add_event_listener(MyParticipantEventHandler(participant_id=participant.id))
def on_participant_left(self, participant: Participant):
print("Participant left:", participant)
Step 4: Listen to Participant Events​
We can listen to participant events as callbacks by inheriting the class ParticipantEventHandler
. We will use MyParticipantEventHandler
class in step 5.
from videosdk import ParticipantEventHandler, Stream
class MyParticipantEventHandler(ParticipantEventHandler):
def __init__(self, participant_id: str):
super().__init__()
self.participant_id = participant_id
def on_stream_enabled(self, stream: Stream):
print("Participant stream enabled:", self.participant_id, stream.kind)
def on_stream_disabled(self, stream: Stream):
print("Participant stream disabled:", self.participant_id, stream.kind)
Step 5: Add Event Listeners​
Now that we have created the event listeners, let's add them to the meeting and participants in the existing main.py
file.
Add the event listener before joining the meeting.
from meeting_events import MyMeetingEventHandler
# Add this line
meeting.add_event_listener(MyMeetingEventHandler())
# Before joining the meeting, add the event listener
meeting.join()
...
Listen for participant events when a participant joins the meeting in the existing meeting_events.py
file.
from participant_events import MyParticipantEventHandler
# Rest of the code as is
def on_participant_joined(self, participant):
print("Participant joined:", participant)
participant.add_event_listener(MyParticipantEventHandler(participant_id=participant.id))
...
Step 6: Run your code​
Run the example using the following command:
python main.py
Output​
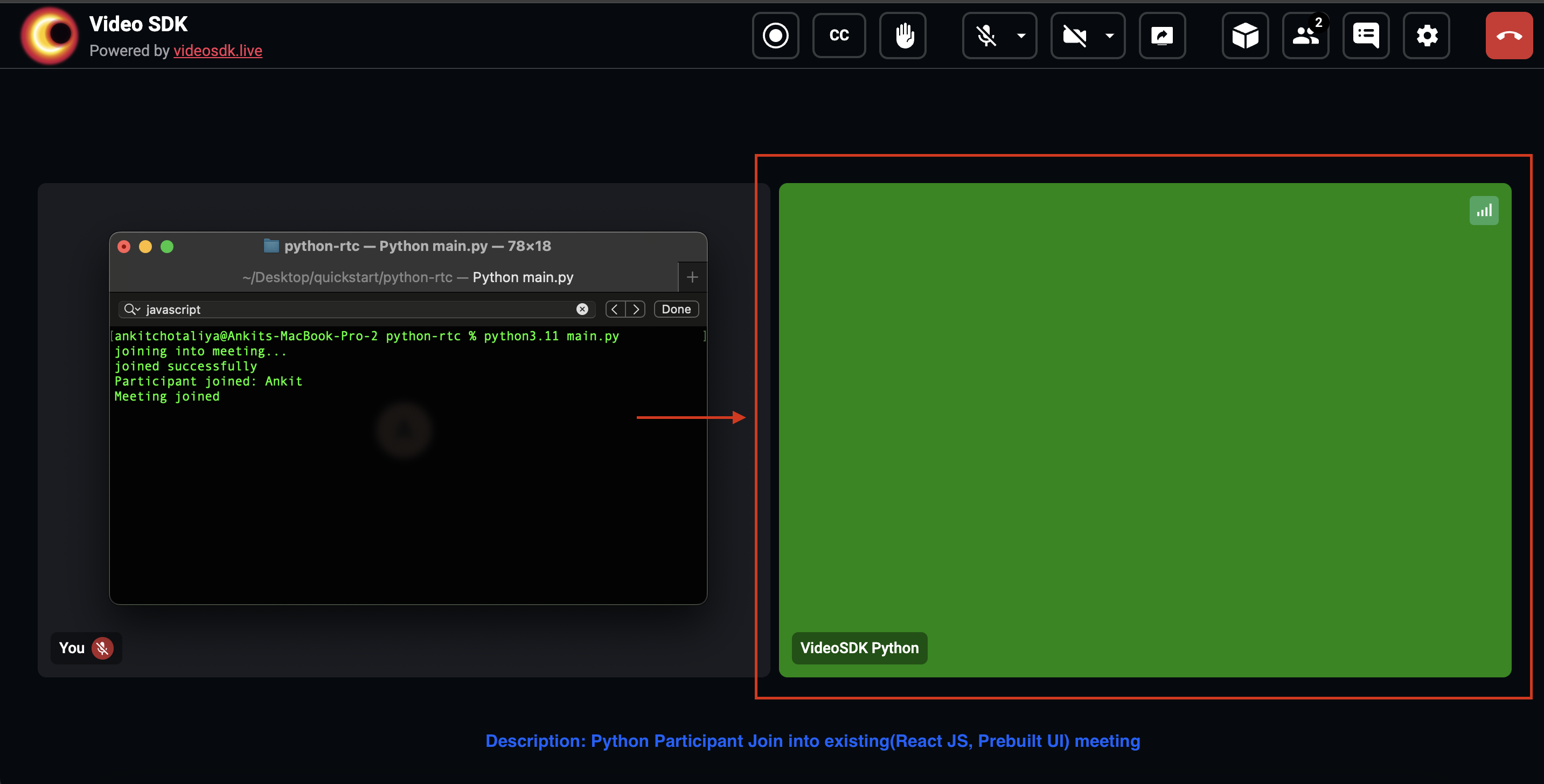
You have completed the implementation of audio-video call using VideoSDK in Python. To explore more features like transform participant video, vision ai go through upcoming detailed guide.
You can check out the complete quick start example here.
Got a Question? Ask us on discord