Rendering Host and Audience Views - React Native
In a live stream setup, only hosts (participants in SEND_AND_RECV
mode) can broadcast their audio and video. Audience members (in RECV_ONLY
mode) are passive viewers who do not share their audio/video.
To ensure optimal performance and a clean user experience, your app should:
- Render video elements only for hosts (i.e., participants in
SEND_AND_RECV
mode). - Display the total audience count to give context on viewership without rendering individual audience tiles.
Filtering and Rendering Hosts​
The steps involved in rendering the video of hosts are as follows.
1. Filtering Hosts and Checking their Mic/Webcam Status
​
In a live stream, only participants in SEND_AND_RECV
mode (i.e., hosts) actively share their audio and video. To render their streams, begin by accessing all participants using the useMeeting
hook. Then, filter out only those in SEND_AND_RECV
mode.
For each of these participants, use the useParticipant
hook, which provides real-time information like displayName, micOn, and webcamOn. Display their name along with the current status of their microphone and webcam. If the webcam is off, show a simple placeholder with their name. If it's on, render their video feed. This ensures only hosts are visible to the audience, keeping the experience clean and intentional.
const LiveStreamView = () => {
const { participants } = useMeeting();
// Filter hosts only
const hostParticipants = [...participants.values()].filter(
(participant) => participant.mode === "SEND_AND_RECV"
);
return (
<View
style={{
flex: 1,
flexDirection: 'row',
flexWrap: 'wrap',
gap: 12,
}}
>
{hostParticipants.map((participant) => (
<ParticipantView key={participant.id} participantId={participant.id} />
))}
</View>
);
};
const ParticipantView = ({ participantId }) => {
const { displayName, micOn, webcamOn, webcamStream } =
useParticipant(participantId);
return (
<View
style={{
height: 250,
backgroundColor: "#404B53",
marginVertical: 8,
}}
>
<Text>{displayName}</Text>
<Text>
Webcam: {webcamOn ? "On" : "Off"} | Mic: {micOn ? "On" : "Off"}
</Text>
</View>
);
};
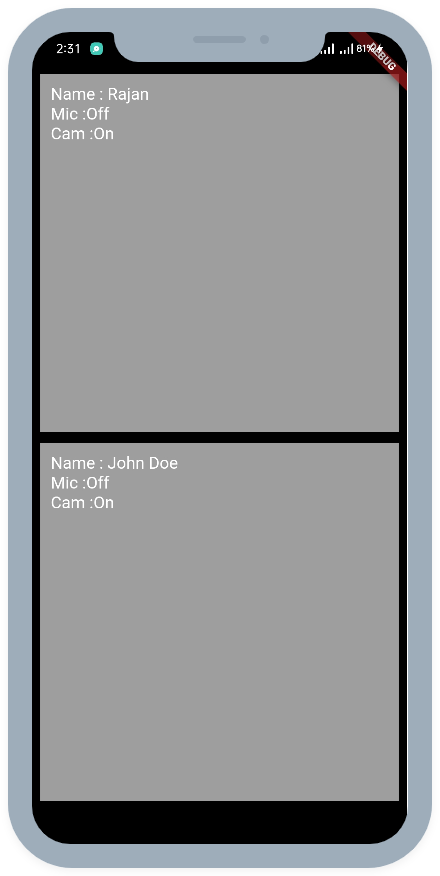
2. Rendering Video Streams of Hosts
​
Once you've filtered for participants in SEND_AND_RECV
mode (i.e., hosts), you can use the useParticipant
hook to access their real-time data, including their webcamStream, webcamOn, and whether they are the local participant.
You can also apply styling to mirror the video for the local participant.
import { useEffect, useRef } from "react";
import { View, Text } from "react-native";
import { useParticipant } from "@videosdk.live/react-native-sdk";
import { RTCView } from "@videosdk.live/react-native-sdk";
const ParticipantView = ({ participantId }) => {
//Getting the webcamStream property
const { webcamOn, displayName, micOn, webcamStream, isLocal } =
useParticipant(participantId);
return (
<View
style={{
height: 250,
backgroundColor: "#404B53",
marginVertical: 8,
padding: 8,
}}
>
<Text style={{ color: "white" }}>{displayName}</Text>
<Text style={{ color: "white" }}>
Webcam:{webcamOn ? "On" : "Off"} Mic: {micOn ? "On" : "Off"}
</Text>
{webcamOn && webcamStream ? (
<RTCView
streamURL={new MediaStream([webcamStream.track]).toURL()}
objectFit={"cover"}
mirror={isLocal ? true : false} //Mirror view for local participant
style={{
height: 200,
}}
/>
) : null}
</View>
);
};
Here, you don't need to render audio separately because RTCView is a component that handles audio stream automatically.
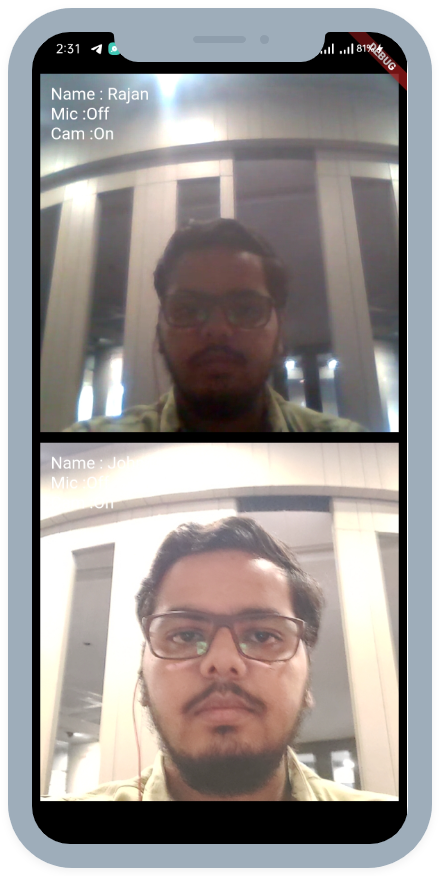
API Reference​
The API references for all the methods and events utilized in this guide are provided below.
Got a Question? Ask us on discord