Invite Guest on Stage - React Native
In this guide, we will see how you can request a viewer to join your livestream by using the changeMode()
.
Before going forward in this guide, do make sure all the attendees join the meeting with mode as SIGNALLING_ONLY
and the host joins with mode as SEND_AND_RECV
Let's first have a look at how we will be using the PubSub
mechanism to acheive the requesting and switching of the participant's mode.
Step 1: Loading Viewer List​
The host will see a list of participants who have joined as SIGNALLING_ONLY
along with a button that, when selected, will invite that user to join the livestream.
import { useMeeting, useParticipant } from "@videosdk.live/react-native-sdk";
import { SafeAreaView, TouchableOpacity, Text, View } from "react-native";
function MeetingView() {
return (
<>
<Text>Viewer List</Text>
<ViewerList />
</>
);
}
function ViewerList() {
const { participants } = useMeeting();
//Filtering only viewer participant
const viewers = [...participants.values()].filter((participant) => {
return participant.mode == Constants.modes.SIGNALLING_ONLY;
});
return (
<SafeAreaView style={{ flex: 1 }}>
{viewers.map((participant) => {
return <ViewerListItem participantId={participant.id} />;
})}
</SafeAreaView>
);
}
function ViewerListItem({ participantId }) {
const { displayName } = useParticipant(participantId);
return (
<View
style={{
flexDirection: "row",
justifyContent: "center",
alignItems: "center",
marginVertical: 8,
}}
>
<Text>{displayName}</Text>
<TouchableOpacity
style={{
marginLeft: 12,
borderWidth: 1,
borderRadius: 12,
padding: 12,
}}
>
<Text>Request to join Livestream</Text>
</TouchableOpacity>
</View>
);
}
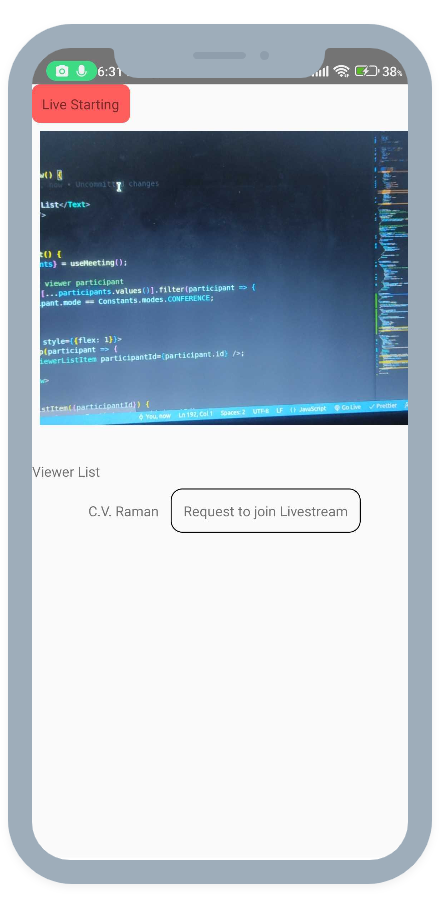
Step 2: Requesting a Viewer to Join Livestream​
We have a Viewer list ready. Now, let us handle the click event for the Join Livestream button.
We will be using CHANGE_MODE_$participantId
as the topic for PubSub.
import { usePubSub } from "@videosdk.live/react-native-sdk";
function ViewerListItem({ participantId }) {
const { displayName } = useParticipant(participantId);
const { publish } = usePubSub(`CHANGE_MODE_${participantId}`);
//Publishing the pubsub message with new mode
const onClickRequestJoinLiveStream = () => {
publish({
mode: "SIGNALLING_ONLY",
});
};
return (
<View
style={{
flexDirection: "row",
justifyContent: "center",
alignItems: "center",
marginVertical: 8,
}}
>
<Text>{displayName}</Text>
<TouchableOpacity
onPress={() => {
onClickRequestJoinLiveStream();
}}
style={{
marginLeft: 12,
borderWidth: 1,
borderRadius: 12,
padding: 12,
}}
>
<Text>Request to join Livestream</Text>
</TouchableOpacity>
</View>
);
}
Step 3: Showing Viewer Request Dialog​
After implementing the Host requesting viewer to join the livestream, let's display the viewer's request dialogue and switch the SIGNALLING_ONLY
mode to SEND_AND_RECV
.
import { usePubSub } from "@videosdk.live/react-native-sdk";
import Video from "react-native-video";
import { Alert } from "react-native";
function ViewerView({}) {
const { hlsState, hlsUrls, localParticipantId, changeMode } = useMeeting();
usePubSub(`CHANGE_MODE_${localParticipantId}`, {
onMessageReceived: (data) => {
const { message, senderName } = data;
if (message.mode === "SEND_AND_RECV") {
showAlert(senderName);
}
},
});
const showAlert = (senderName) => {
Alert.alert(
"Permission",
`${senderName} has requested you to join as a speaker`,
[
{
text: "Reject",
onPress: () => console.log("Cancel Pressed"),
style: "cancel",
},
{
text: "Accept",
onPress: () => {
changeMode("SEND_AND_RECV");
},
},
]
);
};
return (
<SafeAreaView style={{ flex: 1 }}>
{hlsState == "HLS_PLAYABLE" ? (
<>
{/* Render VideoPlayer that will play `downstreamUrl`*/}
<Video
controls={true}
source={{
uri: hlsUrls.downstreamUrl,
}}
resizeMode={"stretch"}
style={{
flex: 1,
backgroundColor: "black",
}}
onError={(e) => console.log("error", e)}
/>
</>
) : (
<SafeAreaView
style={{ flex: 1, justifyContent: "center", alignItems: "center" }}
>
<Text style={{ fontSize: 20 }}>
HLS is not started yet or is stopped
</Text>
</SafeAreaView>
)}
</SafeAreaView>
);
}
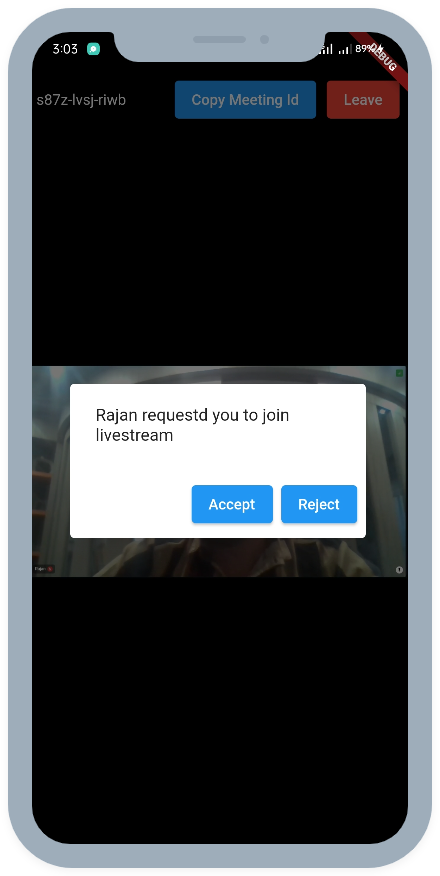
Step 4: Pin the participant​
We need to pin the participant so that he/she can appears on the livestream. To achieve this, we will listen to the callback on the onParticipantModeChanged
of useMeeting
hook.
function MeetingView() {
const mMeeting = useMeeting({});
//We are using a reference to the meeting object because
//While referencing it in the callbacks we want to use its latest state
const mMeetingRef = useRef();
useEffect(() => {
mMeetingRef.current = mMeeting;
}, [mMeeting]);
const { localParticipant, changeMode } = useMeeting({
onParticipantModeChanged: ({ participantId, mode }) => {
const localParticipant = mMeetingRef.current?.localParticipant;
if (participantId == localParticipant.id) {
if (mode == "SEND_AND_RECV") {
localParticipant.pin();
} else {
localParticipant.unpin();
}
}
},
});
return <>...</>;
}
If you want complete source code, you can checkout our code sample
API Reference​
The API references for all the methods utilized in this guide are provided below.
Got a Question? Ask us on discord