Start or Join Room - Flutter
After the successful installation of VideoSDK, the next step is to integrate VideoSDK features with your webApp/MobileApp.
To Communicate with other participant's audio or video call, you will need to join the room.
This guide will provide an overview of how to configure, initialize and join a VideoSDK room.
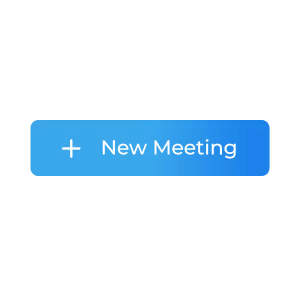
1. Configuration​
To configure a room, you will need generated token and roomId, we had discussed in Server Setup. This code snippet calls API from local server
Scenario 1 - Suppose you don't have any roomId, you can simply generate roomId by invoking create-room
API.
Scenario 2 - Suppose you have roomId, now you don't have to call create-room
API to generate roomId, instead you can call validate-room
API to validate roomId.
Token generation API is necessary for both scenario.
import 'dart:convert';
import 'package:http/http.dart' as http; // For API Calling, you need to add third party package "http"
import 'package:videosdk/videosdk.dart';
// States Defined in Stateful Component.
String? roomId;
String? token;
void _getRoomIdAndToken() async {
final LOCAL_SERVER_URL = dotenv.env['LOCAL_SERVER_URL'];
// Calling get-token API.
final Uri tokenUrl = Uri.parse('$LOCAL_SERVER_URL/get-token');
final http.Response tokenResponse = await http.get(tokenUrl);
final dynamic _token = json.decode(tokenResponse.body)['token'];
// Calling create-room API.
final Uri roomIdUrl =
Uri.parse('$LOCAL_SERVER_URL/create-room/');
final http.Response roomIdResponse =
await http.post(roomIdUrl, body: {"token": _token});
final _roomId = json.decode(roomIdResponse.body)['roomId'];
// Setting into states of stateful widget
setState(() {
token = _token;
roomId = _roomId;
});
}
// This API is for validate the room id
// Not require to call this API after create room API
dynamic validateRoom(token, roomId) async {
final String LOCAL_SERVER_URL = dotenv.env['LOCAL_SERVER_URL'];
final Uri validateRoomUrl =
Uri.parse('$LOCAL_SERVER_URL/validate-room/$roomId');
final http.Response validateRoomResponse =
await http.post(validateRoomUrl, body: {"token": token});
final _roomId = json.decode(validateRoomResponse.body)['roomId'];
if (_roomId != null) {
return _roomId;
} else {
return null;
}
}
2. Initialization​
After configuration, you will have to Initialize
room by providing name, roomId, micEnabled, camEnabled & maxResolution.
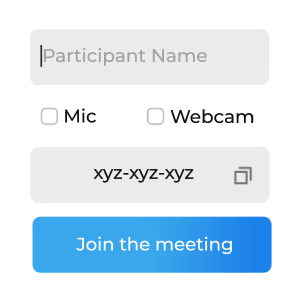
import 'package:flutter/material.dart';
import 'package:videosdk/videosdk.dart';
class MyApp extends StatelessWidget {
final Room = VideoSDK.createRoom(
roomId: "<Id-of-Room>",
displayName: "<Name-of-participant>",
micEnabled: "<Flag-to-enable-mic>",
camEnabled: "<Flag-to-enable-cam>",
token: "<Authentication-token>",
notification: const NotificationInfo(
title: "Video SDK",
message: "Video SDK is sharing screen",
icon: "notification_share",
),
);
@override
Widget build(BuildContext context) {
return return Container(); // Returning widget
}
}
3. Join​
After configuration & initialization, the third step is to call join() to join a room.
After joining, you will be able to Manage Participant in a room.
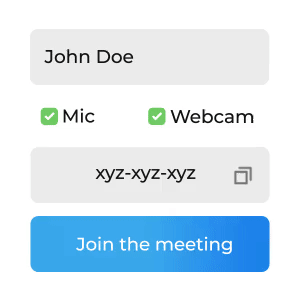
// Join the room
room?.join();
Events​
Following events are emitted on the room
when it is successfully joined.
- Local Participant will receive a
roomJoined
event when successfully joined. - Remote Participant will receive a
participantJoined
event with the newly joinedParticipant
object from the event callback.
room.on(Events.roomJoined, () => {
print("Room Joined Successfully");
});
room.on(Events.participantJoined, (participant) => {
print("New Participant Joined");
});
Got a Question? Ask us on discord