Start or Join Meeting - Flutter
After the successful installation of VideoSDK, the next step is to integrate VideoSDK features with your webApp/MobileApp.
To Communicate with other participant's audio or video call, you will need to join the meeting.
This guide will provide an overview of how to configure, initialize and join a VideoSDK meeting.
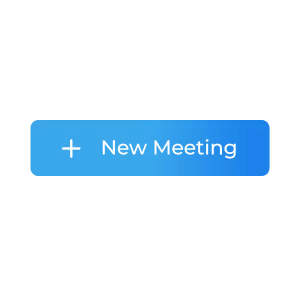
1. Configuration​
To configure a meeting, you will need generated token and meetingId, we had discussed in Server Setup. This code snippet calls API from local server
Scenario 1 - Suppose you don't have any meetingId, you can simply generate meetingId by invoking create-meeting
API.
Scenario 2 - Suppose you have meetingId, now you don't have to call create-meeting
API to generate meetingId, instead you can call validate-meeting
API to validate meetingId.
Token generation API is necessary for both scenario.
import 'dart:convert';
import 'package:http/http.dart' as http; // For API Calling, you need to add third party package "http"
import 'package:videosdk/rtc.dart';
// States Defined in Stateful Component.
String? meetingId;
String? token;
void _getMeetingIdAndToken() async {
final LOCAL_SERVER_URL = dotenv.env['LOCAL_SERVER_URL'];
// Calling get-token API.
final Uri tokenUrl = Uri.parse('$LOCAL_SERVER_URL/get-token');
final http.Response tokenResponse = await http.get(tokenUrl);
final dynamic _token = json.decode(tokenResponse.body)['token'];
// Calling create-meeting API.
final Uri meetingIdUrl =
Uri.parse('$LOCAL_SERVER_URL/create-meeting/');
final http.Response meetingIdResponse =
await http.post(meetingIdUrl, body: {"token": _token});
final _meetingId = json.decode(meetingIdResponse.body)['meetingId'];
// Setting into states of stateful widget
setState(() {
token = _token;
meetingId = _meetingId;
});
}
// This API is for validate the meeting id
// Not require to call this API after create meeting API
dynamic validateMeeting(token, meetingId) async {
final String LOCAL_SERVER_URL = dotenv.env['LOCAL_SERVER_URL'];
final Uri validateMeetingUrl =
Uri.parse('$LOCAL_SERVER_URL/validate-meeting/$meetingId');
final http.Response validateMeetingResponse =
await http.post(validateMeetingUrl, body: {"token": token});
final _meetingId = json.decode(validateMeetingResponse.body)['meetingId'];
if (_meetingId != null) {
return _meetingId;
} else {
return null;
}
}
2. Initialization​
After configuration, you will have to Initialize
meeting by providing name, meetingId, micEnabled, webcamEnabled & maxResolution.
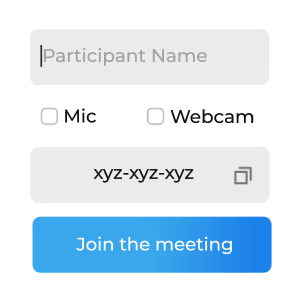
import 'package:flutter/material.dart';
import 'package:videosdk/rtc.dart';
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MeetingBuilder(
meetingId: "<Id-on-meeting>",
displayName: "<Name-of-participant>",
micEnabled: "<Flag-to-enable-mic>",
webcamEnabled: "<Flag-to-enable-webcam>",
token: "<Authentication-token>",
builder: (Meeting: meeting) {
// Do Something
return Container(); // Returning widget
}
);
}
}
3. Join​
After configuration & initialization, the third step is to call join() to join a meeting.
After joining, you will be able to Manage Participant in a meeting.
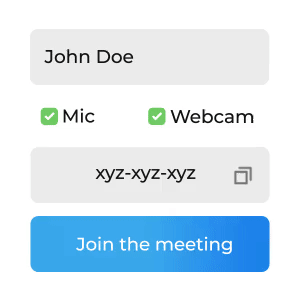
// Join the meeting
meeting?.join();
Got a Question? Ask us on discord