Setup HLS Player - React Native
This guide focuses on creating the Component responsible for playing the HLS stream. Before proceeding, ensure that you have set up a VideoSDK meeting, enabling you to join the room. For instructions on setting up a VideoSDK meeting, refer to the quick start guide.
For playing the HLS stream, you need to use the react-native-video library.
Before initiating a live stream, ensure that HLS is running using startHLS; otherwise, downstream won't be available.
1. Setup Component with HLS events
​
Step 1:
Begin by creating a new component named HLSPlayer
. Place this component inside the MeetingProvider
to ensure access to the VideoSDK hooks.
import { SafeAreaView } from "react-native";
function Container() {
return <HLSPlayer />;
}
function HLSPlayer() {
return (
<SafeAreaView style={{ flex: 1, backgroundColor: "black" }}> </SafeAreaView>
);
}
Step 2:
Now, incorporate the placeholder that will be displayed when there is no active HLS. Utilize the hlsState
from the useMeeting
hook to determine the presence of an active HLS.
import { useMeeting, Constants } from "@videosdk.live/react-native-sdk";
import { SafeAreaView, Text } from "react-native";
function HLSPlayer() {
const { hlsUrls, hlsState } = useMeeting();
return (
<SafeAreaView style={{ flex: 1, backgroundColor: "black" }}>
{hlsState == "HLS_PLAYABLE" ? (
<>
<Text>We will setup player here</Text>
</>
) : (
<SafeAreaView
style={{ flex: 1, justifyContent: "center", alignItems: "center" }}
>
<Text style={{ fontSize: 20, color: "white" }}>
HLS is not started yet or is stopped
</Text>
</SafeAreaView>
)}
</SafeAreaView>
);
}
2. Playing HLS stream
​
Step 1:
To play the HLS stream, you need to have the react-native-video library. To install the library, use the following command.
npm install react-native-video
Step 2:
Next step is to add a Video
component that will play the livestream.
// imports react-native-video
import Video from "react-native-video";
function HLSPlayer() {
const { hlsUrls, hlsState } = useMeeting();
return (
<SafeAreaView style={{ flex: 1, backgroundColor: "black" }}>
{hlsState == "HLS_PLAYABLE" ? (
{/* Render VideoPlayer that will play `downstreamUrl`*/}
<Video
controls={true}
source={{
uri: hlsUrls.downstreamUrl,
}}
resizeMode={"stretch"}
style={{
flex: 1,
backgroundColor: "black",
}}
onError={(e) => console.log("error", e)}
/>
) : (
<SafeAreaView
style={{ flex: 1, justifyContent: "center", alignItems: "center" }}
>
<Text style={{ fontSize: 20, color: "white" }}>
HLS is not started yet or is stopped
</Text>
</SafeAreaView>
)}
</SafeAreaView>
);
}
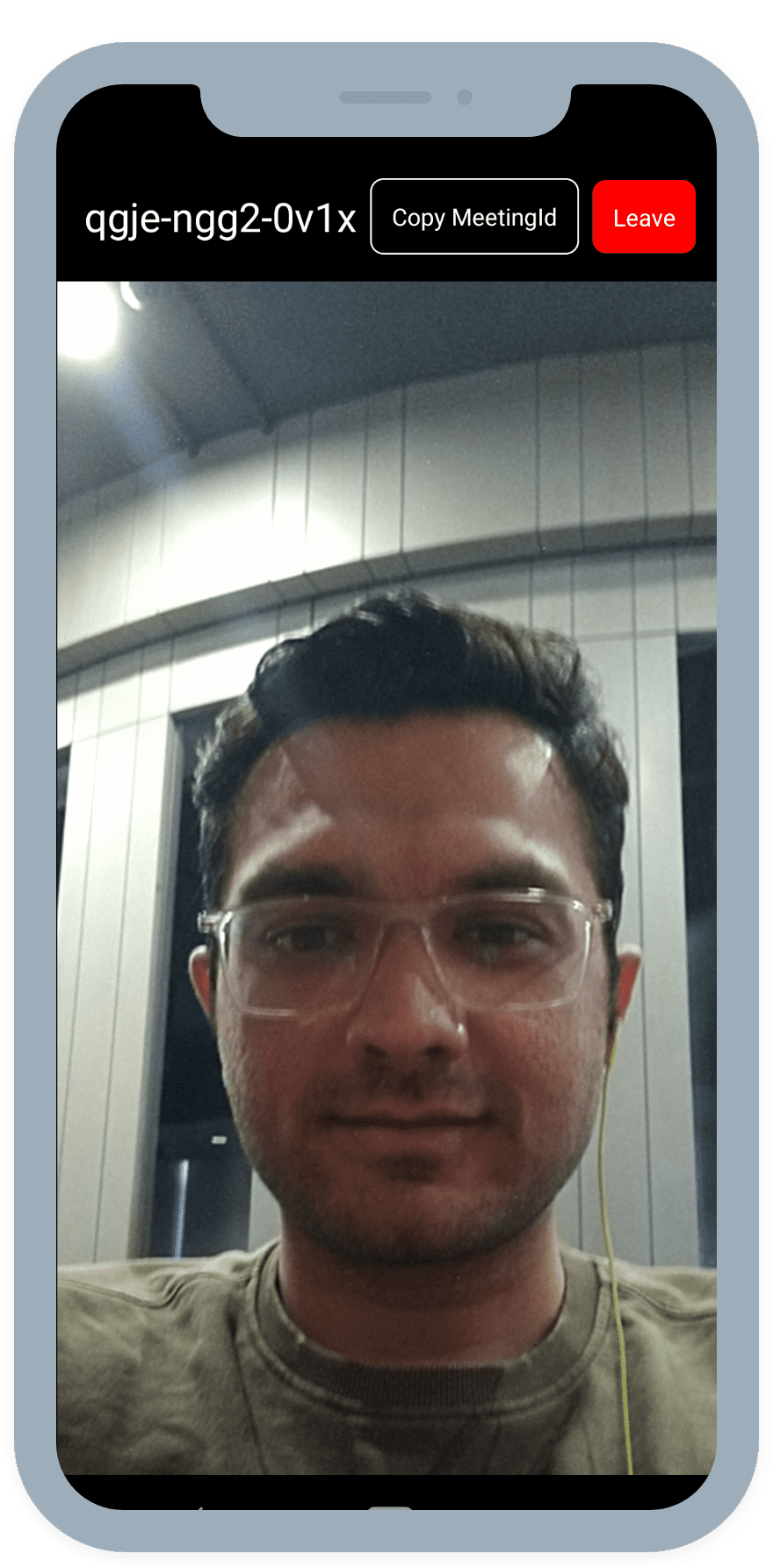
API Reference​
The API references for all the methods utilized in this guide are provided below.
Got a Question? Ask us on discord